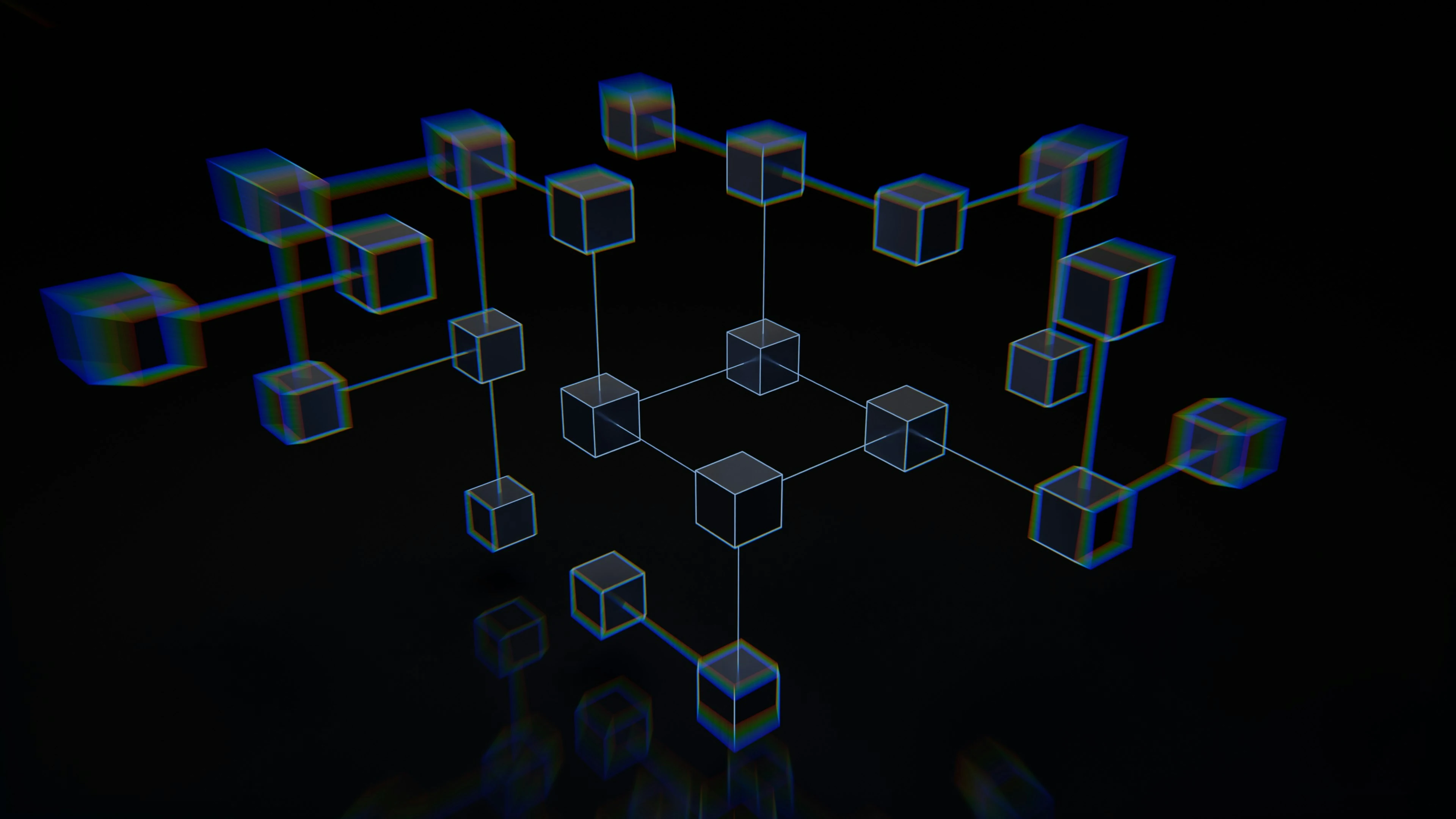
Don't ForgetReactCheat Sheet
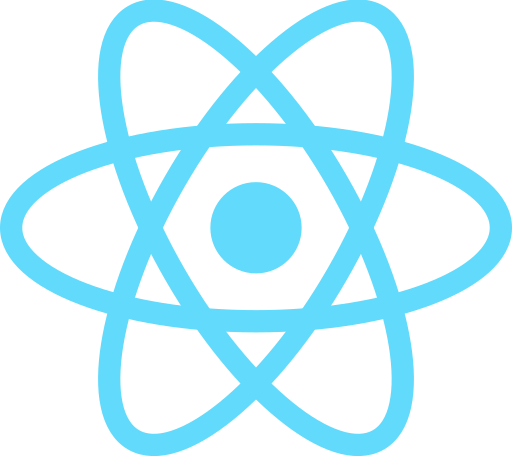
Getting Started
Everything you need to start building with React
React Fundamentals
Core concepts you need to understand React
Advanced Concepts
Take your React skills to the next level
React Ecosystem
Popular tools and frameworks in the React ecosystem
Getting Started
Everything you need to start building with React
When to Use React?
React isn't always the best choice. Here's a comparison with alternatives:
REACT USE CASES
- →Websites that need to update content without refreshing the page
- →Apps with lots of reusable pieces (like buttons, cards, forms)
- →When you want to use pre-made components from other developers (libraries)
- →When your team knows JavaScript well
- →When you want to build a professional web app
VS VANILLA JAVASCRIPT
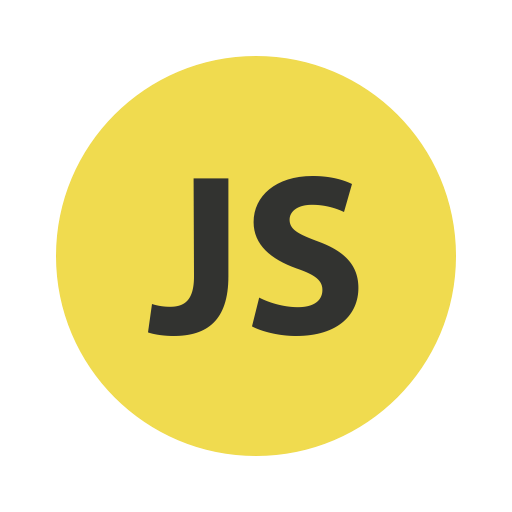
Simple websites, landing pages, or when you're learning web development basics
Pros:
- Works directly in the browser
- Can use without external libraries
- Can be faster
Cons:
- Harder to re-use code
- Harder to keep code organized
- More manual work
VS VUE.JS
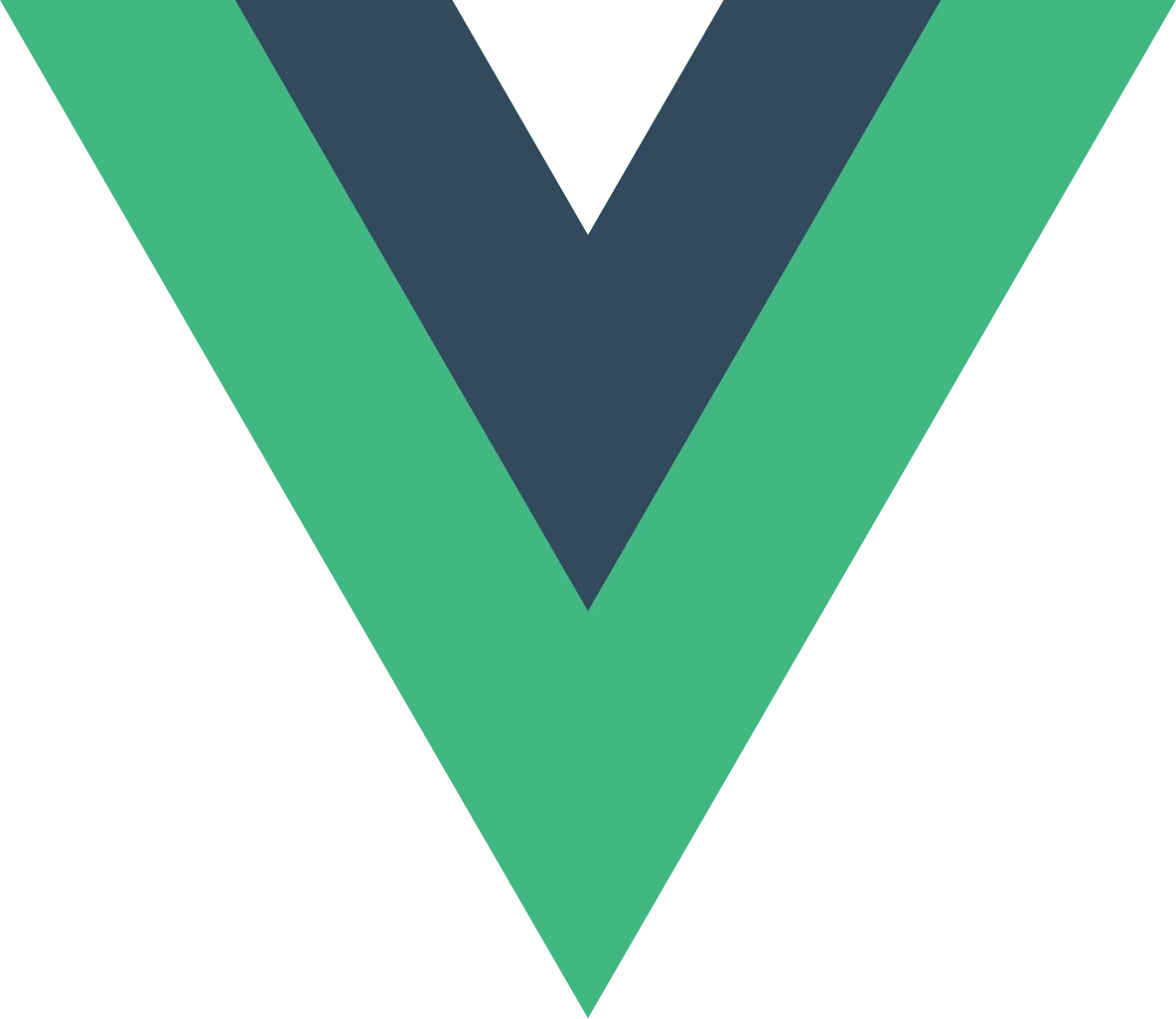
Smaller projects, or when you want an easier learning curve
Pros:
- Easier to learn than React
- Good documentation
- HTML-like syntax
Cons:
- Fewer job opportunities
- Fewer ready-made components
- Less popular in big companies
VS SVELTE
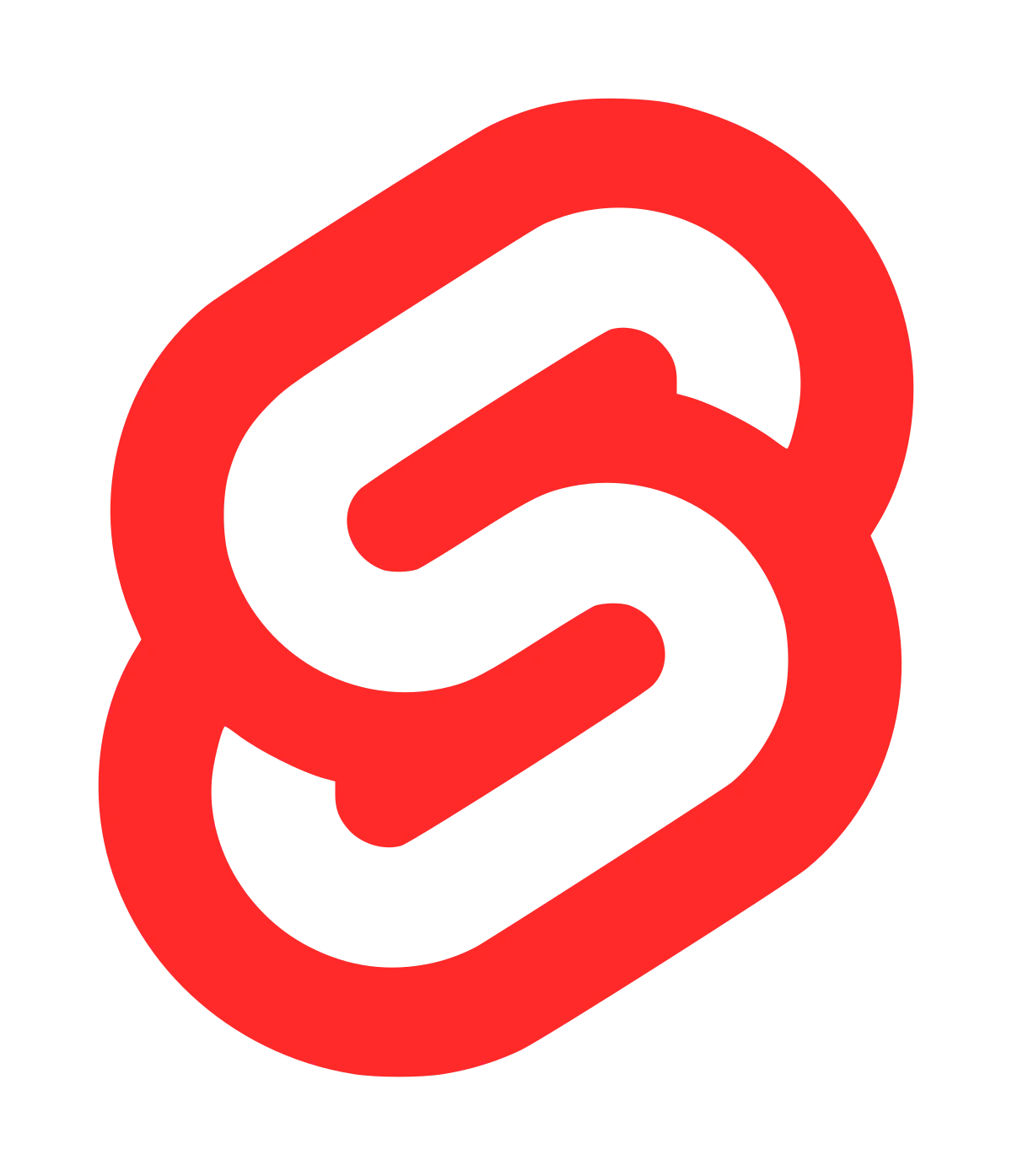
Small to medium projects, or when website speed is very important
Pros:
- Less code to write
- Faster websites
- Simple to understand
Cons:
- Newer and less popular
- Harder to find help
- Fewer tutorials
Setting Up React
Everything you need to start your first React project
WHAT YOU'LL NEED
Node.js & npm
Node.js includes npm (Node Package Manager)
- Download Node.js from nodejs.org
- This automatically installs npm
- Verify with 'node --version' and 'npm --version' in terminal
RECOMMENDED APPROACH
- →Frameworks handle complex setup for you
- →They include routing, optimization, and other features
- →Better developer experience out of the box
- →Industry standard approach
- →Better documentation and community support
QUICK START
# Create new Next.js project (recommended)
npx create-next-app@latest my-app
# Navigate to project
cd my-app
# Install dependencies
npm install
# Start development server
npm run dev
ADDING LIBRARIES
# Install a single library
npm install react-icons
# Install multiple libraries
npm install axios react-query @mui/material
UNDERSTANDING THE STRUCTURE
my-react-app/
├── node_modules/ # Dependencies
├── public/ # Static files
├── src/ # Your code goes here
│ ├── app/ # Your pages (in Next.js)
│ └── components/# Reusable components
├── package.json # Project configuration
└── README.md # Documentation
React Fundamentals
Core concepts you need to understand React
JSX
Almost like HTML, with a few key differences
CLASS BECOMES CLASSNAME
// In HTML:
<p class="primary-text"></p>
// In React:
<p className="primary-text"></p>
STYLE SYNTAX
// In HTML:
<p style="color: white"></p>
// In React:
<p style={{ color: "white" }}></p>
COMPONENTS
function MyComponent() {
return <p>This is JSX!</p>
}
EVENT HANDLERS
function MyComponent() {
return (
<form onSubmit={...}>
<input type="text" onChange={...} />
<button onClick={...}>Click me!</button>
<input type="submit" />
</form>
)
}
EVENT HANDLER SYNTAX
// ❌ This doesn't work right
<button
onClick={console.log("clicked")}
>
Click me!
</button>
// ✅ This does (note anonymous function)
<button
onClick={() => console.log("clicked")}
>
Click me!
</button>
EVENT OBJECT USAGE
<input
type="text"
onChange={e => console.log
(e.target.value)} />
logs everytime we type
EVENT OBJECT OPTIONAL
<button
onClick={() => setClicked(true)}
>
Click me
</button>
sets state variable on click
Components
Think of Components as "Custom JSX Elements" that we can define, and then use one or more times. Ultimately, the components are just like variables which will be converted to HTML.
COMPONENT DEFINITION
// MyComponent.js
export default function MyComponent() {
return <p>This is JSX!</p>
}
COMPONENT USAGE
// App.js
import MyComponent from "./MyComponent";
export default function App() {
return (
<>
<MyComponent />
<MyComponent />
<MyComponent />
</>
);
}
HTML OUTPUT
<!-- index.html -->
<p>This is JSX!</p>
<p>This is JSX!</p>
<p>This is JSX!</p>
COMPONENT SIZE
- →Will this component be used more than once?
- →Does it make sense as a logical grouping? Ex. a navigation bar, or page layout
- →Does it handle logic, or just display data?
The Component Tree
The convention is 1 component per file. We MUST export components to use them in other files
EXPORT COMPONENT
export default function MyComponent() { ... }
IMPORT COMPONENT
import MyComponent from "./MyComponent";
COMPONENT COMPOSITION
export default function AllMyComponents() {
return (
<>
<MyComponent />
<MyComponent />
<MyComponent />
</>
)
}
Props
The "arguments" of a component, they're how we pass data from one component to another.
PASSING PROPS
<MyComponent name="Aaron" />
RECEIVING PROPS
function MyComponent(props) {
console.log(props.name);
// Aaron prints
}
DESTRUCTURING PROPS
function MyComponent({ name }) {
return <p>Hello, {name}</p>
}
PROPS IN PRACTICE
// React
function AllMyComponents() {
return (
<div>
<MyComponent name="Aaron" />
<MyComponent name="Jack" />
<MyComponent name="Jan" />
</div>
)
}
// HTML Result
<div>
<p>Hello, Aaron</p>
<p>Hello, Jack</p>
<p>Hello, Jan</p>
</div>
SAVING TIME WITH .MAP
const names = ["Aaron", "Jack", "Jan"];
function AllMyComponents() {
return (
<div>
{names.map(name => (
<MyComponent key={name} name={name} />
))}
</div>
)
}
// Same HTML Result
<div>
<p>Hello, Aaron</p>
<p>Hello, Jack</p>
<p>Hello, Jan</p>
</div>
State & State Hook
The "internal variables" of a component.
DECLARING STATE
import React from 'react'
function MyComponent() {
const [myName, setMyName] =
React.useState("Aaron")
...
}
UPDATING STATE
setMyName("Jack"); // correct
myName = "jack"; // won't work
STATE WITH ARRAYS
const [myNames, setMyNames] =
React.useState(["Aaron"]);
// won't work
myNames.push("Jack")
// works, creates a copy with array
// spreading, adds "Jack" at the end
setMyNames([...myNames, "Jack"]);
Styling With React
In React, you can still use CSS files, for sure. But Styled Components are more popular, especially with UI component libraries (see next section).
BASIC STYLED COMPONENT
const Block = styled.div`
margin: 10px;
padding: 10px;
`;
EXTENDING STYLES
const SmallBlock = styled(Block)`
width: 100px;
`
const BigBlock = styled(Block)`
width: 200px;
`
STYLED COMPONENTS USAGE
// Using the components
<SmallBlock>Small content</SmallBlock>
<BigBlock>Larger content</BigBlock>
Advanced Concepts
Take your React skills to the next level
useEffect & React Life Cycle
When components get added to the page, they start the "component life cycle" There are 3 key events: Mount, Update, UnMount
LIFECYCLE
Component is added to DOM. useEffect with empty [] runs here.
Props or state change. useEffect with dependencies runs here.
Component is removed. useEffect cleanup functions run here.
BASIC USEEFFECT
import React from 'react'
function MyComponent() {
React.useEffect(() => {
fetch("https://myserverurl.com/items")
.then(...)
}, [])
}
DEPENDENCIES ARRAY
React.useEffect(() => {
fetch("https://myserverurl.com/items/" + itemID)
.then(...)
}, [itemID])
CLEANUP FUNCTION
React.useEffect(() => {
return () => alert("goodbye!")
}, [])
React Router
React Router enables us to do "Client Side Routing", so we can have some navigation in our React apps with multiple pages and paths that can be observed in the URL bar.
HTML VS REACT ROUTING
// index.html
<h1>Hello there!</h1>
<a href="faq.html">Go to FAQ</a>
// faq.html
<h1>FAQ</h1>
<a href="index.html">Go to home</a>
REACT ROUTER SETUP
import {
BrowserRouter,
Routes,
Route,
} from "react-router-dom";
import Home from "./Home"
import Faq from "./Faq"
function App() {
return(
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="expenses" element={<Faq />} />
</Routes>
</BrowserRouter>
);
}
ROUTER LINKS
// Home.js
import { Link } from "react-router-dom";
export default function Home() {
return(
<>
<h1>Hello there!</h1>
<Link to="/faq">Go to FAQ</Link>
</>
);
}
// Faq.js
import { Link } from "react-router-dom";
export default function Faq() {
return(
<>
<h1>FAQ</h1>
<Link to="/">Go to Home</Link>
</>
);
}
Context & Provider
Share state between components without prop drilling. Context provides a way to pass data through the component tree without having to pass props manually at every level.
CREATING CONTEXT
// ThemeContext.js
import { createContext } from 'react';
export const ThemeContext = createContext();
export function ThemeProvider({ children }) {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
}
USING CONTEXT
// App.js
import { ThemeProvider } from './ThemeContext';
function App() {
return (
<ThemeProvider>
<Header />
<MainContent />
<Footer />
</ThemeProvider>
);
}
CONSUMING CONTEXT
// DeepNestedComponent.js
import { useContext } from 'react';
import { ThemeContext } from './ThemeContext';
function DeepNestedComponent() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<button onClick={() => setTheme(
theme === 'light' ? 'dark' : 'light'
)}>
Current theme: {theme}
</button>
);
}
CONTEXT VS PROPS
- →Use Context for global state (theme, user auth, language)
- →Use Props for component-specific data
- →Context can make components less reusable
- →Don't overuse - Context changes trigger re-renders
Debugging React
Common debugging techniques and tools to find and fix problems in your React apps
REACT DEVELOPER TOOLS
- →Inspect component props and state
- →See component hierarchy
- →Track component re-renders
- →Debug performance issues
- →View source locations
COMMON ERROR MESSAGES
// Objects are not valid as React child
return <div>{myObject}</div> // ❌
return <div>{JSON.stringify(myObject)}</div> // ✅
// Cannot update a component while rendering
function Bad() {
const [count, setCount] = useState(0)
setCount(count + 1) // ❌ Infinite loop!
}
// Missing dependencies in useEffect
useEffect(() => {
console.log(data) // ESLint warning
}, []) // Missing 'data' dependency
CONSOLE DEBUGGING
function MyComponent({ data }) {
console.log('Component rendered:', { data })
useEffect(() => {
console.log('Effect ran:', { data })
return () => console.log('Cleanup:', { data })
}, [data])
return <div>{/* ... */}</div>
}
Deploying React Apps
Learn how to put your React app on the internet
BUILD YOUR APP
# Create production build
npm run build
# Preview build locally
npm run preview
ON VERCEL
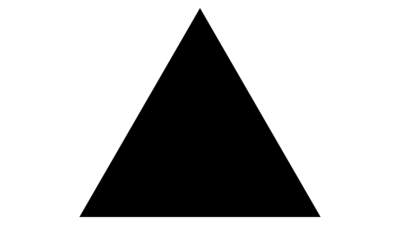
Key Features
- Best option for Next.js projects
- Automatic deployments from Git
- Built-in analytics and monitoring
- Great developer experience
ON NETLIFY
Key Features
- Great for static React apps
- Simple Git integration
- Includes CDN and SSL
- Form handling built-in
- Free tier available
ON GITHUB PAGES
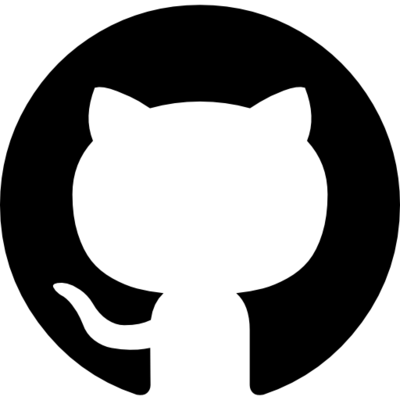
Key Features
- Free hosting for public repos
- Direct integration with GitHub
- Manual deployment process
- Good for personal projects
- Requires some configuration
TypeScript with React
TypeScript helps catch errors before they happen by adding types to your code
COMPONENT PROPS
// Define the shape of your props
type ButtonProps = {
text: string
onClick: () => void
color?: 'primary' | 'secondary'
}
// Use them in your component
function Button({
text,
onClick,
color = 'primary'
}: ButtonProps) {
return (
<button
onClick={onClick}
className={color}
>
{text}
</button>
)
}
TYPING HOOKS
// State with type
const [user, setUser] = useState<User | null>(null)
// Event with type
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
setUser(e.target.value)
}
Common React Patterns
Frequently used patterns and solutions in React applications
LAYOUT PATTERN
// Layout.js
export default function Layout({ children }) {
return (
<div>
<nav>{/* Navigation */}</nav>
<main>{children}</main>
<footer>{/* Footer */}</footer>
</div>
)
}
// Page.js
export default function Page() {
return (
<Layout>
<h1>My Page Content</h1>
</Layout>
)
}
AUTH PATTERN
function PrivateRoute({ children }) {
const { user } = useAuth()
if (!user) {
return <Navigate to="/login" />
}
return children
}
// Usage
<PrivateRoute>
<SecretPage />
</PrivateRoute>
LOADING STATES
function ProductList() {
const [isLoading, setIsLoading] =
useState(true)
const [error, setError] = useState(null)
const [data, setData] = useState([])
if (isLoading) return <Spinner />
if (error) return <Error message={error} />
return <div>{/* Show data */}</div>
}
Common React Mistakes
Learn from others' mistakes - avoid these common pitfalls when building React apps
STATE MISTAKES
// ❌ Modifying state directly
const [user, setUser] = useState({ name: 'John' })
user.name = 'Jane' // Wrong!
// ✅ Using setState correctly
setUser({ ...user, name: 'Jane' }) // Correct!
// ❌ Using state value right after setting it
setCount(count + 1)
console.log(count) // Still shows old value!
// ✅ Use useEffect to react to changes
useEffect(() => {
console.log(count) // Shows new value
}, [count])
USEEFFECT MISTAKES
// ❌ Missing dependencies
useEffect(() => {
setUser(data)
}, []) // Warning: 'data' is missing!
// ✅ Including all dependencies
useEffect(() => {
setUser(data)
}, [data])
// ❌ Infinite loop
useEffect(() => {
setCount(count + 1)
}) // No dependency array = runs every render!
// ✅ Controlled updates
useEffect(() => {
setCount(count + 1)
}, [someValue])
RENDER MISTAKES
// ❌ Creating components inside components
function ParentComponent() {
// This creates a new ChildComponent every render!
function ChildComponent() { return <div>Child</div> }
return <ChildComponent />
}
// ✅ Define components outside
function ChildComponent() { return <div>Child</div> }
function ParentComponent() {
return <ChildComponent />
}
PROPS MISTAKES
// ❌ Modifying props
function Child(props) {
props.value = 123 // Never modify props!
}
// ✅ Use state if you need to modify values
function Child(props) {
const [value, setValue] = useState(props.value)
}
// ❌ Not handling null/undefined props
function Profile({ user }) {
return <h1>{user.name}</h1> // Crashes if user is null!
}
// ✅ Always handle edge cases
function Profile({ user }) {
if (!user) return <div>Loading...</div>
return <h1>{user.name}</h1>
}
KEY MISTAKES
// ❌ Using index as key in dynamic lists
{items.map((item, index) => (
<Item key={index} /> // Bad for lists that change!
))}
// ✅ Using unique, stable IDs
{items.map((item) => (
<Item key={item.id} />
))}
EVENT HANDLER MISTAKES
// ❌ Calling function instead of passing it
<button onClick={handleClick()}> {/* Wrong! */}
// ✅ Passing the function reference
<button onClick={handleClick}> {/* Correct! */}
// ❌ Not preventing form submit default
<form onSubmit={handleSubmit}>
// ✅ Preventing default behavior
<form onSubmit={(e) => {
e.preventDefault()
handleSubmit()
}}>
CONDITIONAL RENDERING MISTAKES
// ❌ Using statements in JSX
return (
<div>
{if (condition) { // Won't work!
return <span>Hi</span>
}}
</div>
)
// ✅ Using expressions instead
return (
<div>
{condition && <span>Hi</span>}
{condition ? <span>Hi</span> : <span>Bye</span>}
</div>
)
React Ecosystem
Popular tools and frameworks in the React ecosystem
React Frameworks
Modern React development often uses a framework. Here are the most popular options:
NEXT.JS
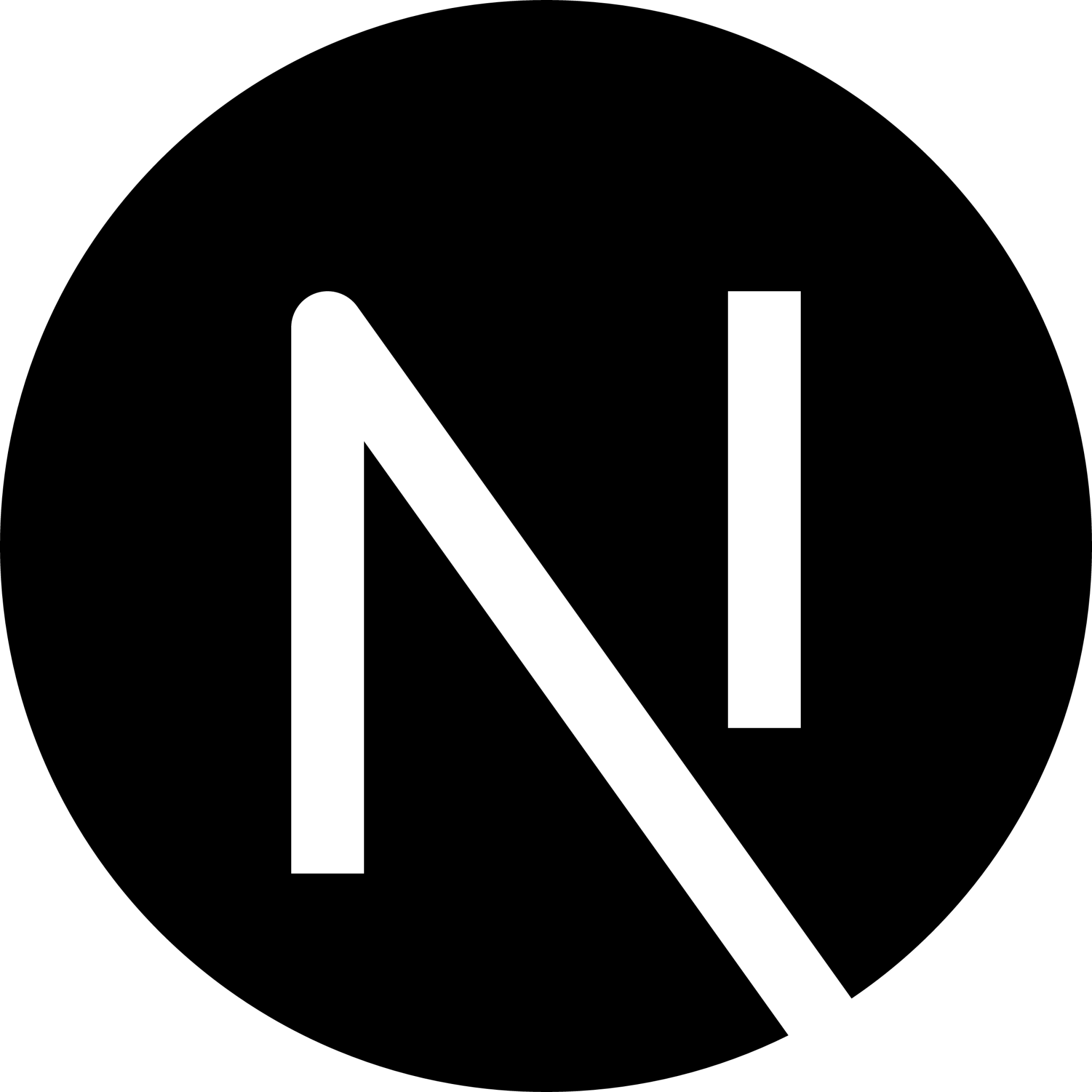
Professional websites that need good performance and SEO
Pros:
- Pre-built or dynamic pages
- Easy Vercel deployment
- Good for SEO
Cons:
- Strict rules & structure
- Moderate learning curve
REMIX
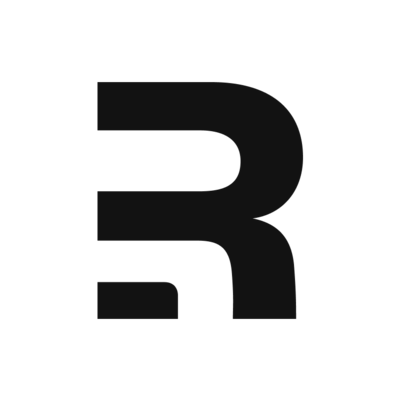
Web apps that need to work well even with slow internet
Pros:
- Good error handling
- Works without JavaScript
- Fast page loads
Cons:
- Very new
- Fewer tutorials available
- Different mental model
GATSBY
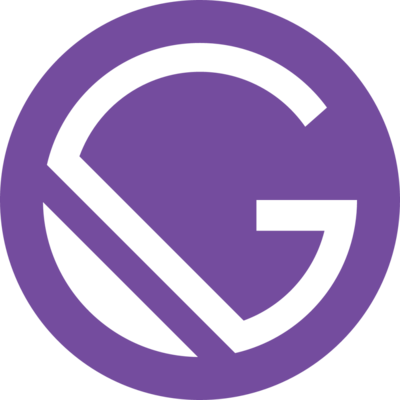
Blogs, marketing sites, and content-heavy websites
Pros:
- Fast static websites
- Great for blogs
- Lots of plugins
Cons:
- Slow to build
- Needs GraphQL knowledge
- Not great for dynamic content
VITE
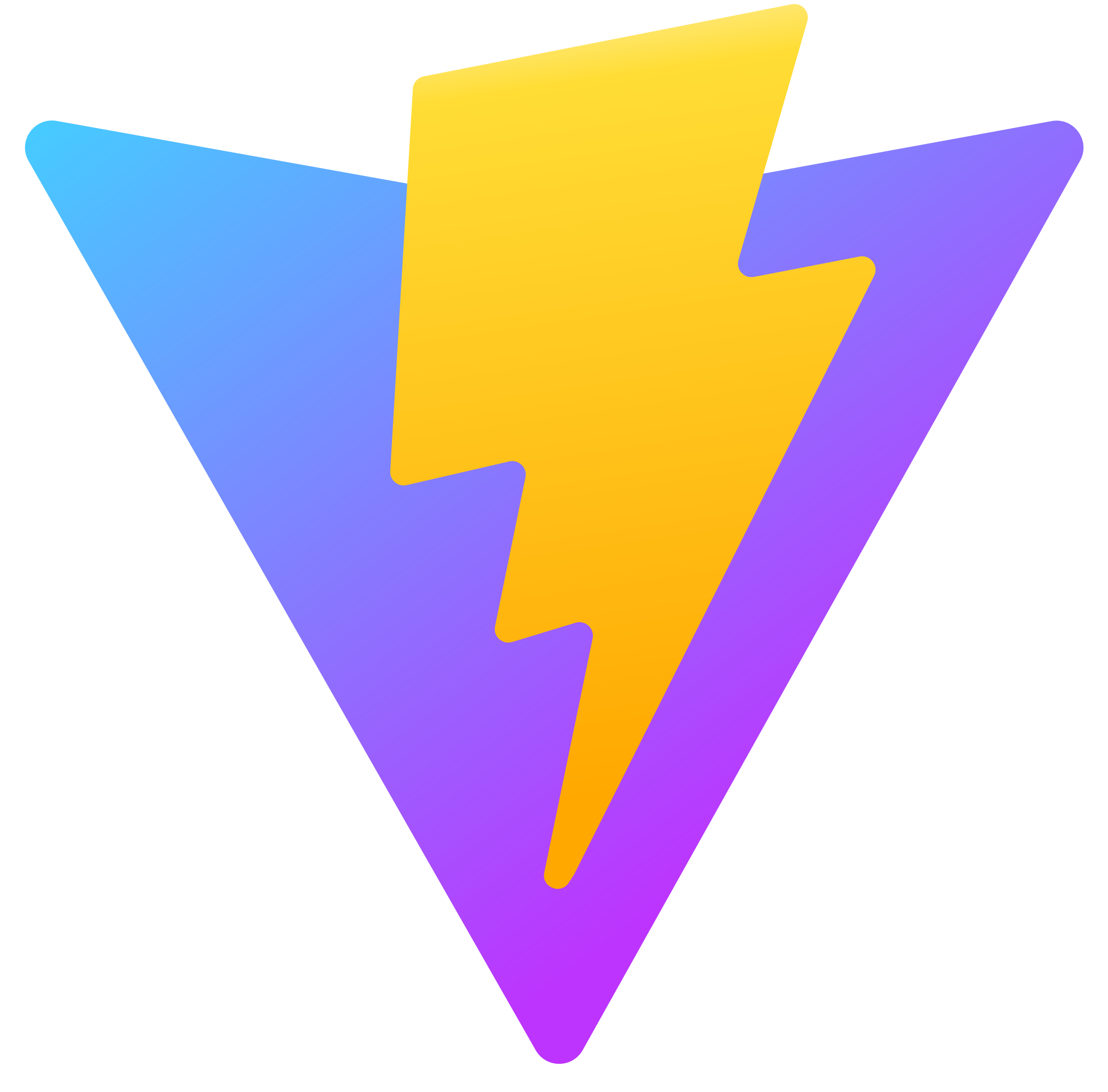
When you want a simple, fast, minimal way to start a React project
Pros:
- Very fast development
- Easy to set up
- Modern features
Cons:
- Less features out of the box
- More setup required
Popular React Libraries
The React ecosystem is rich with libraries that solve common problems. Here are some of the most popular ones: