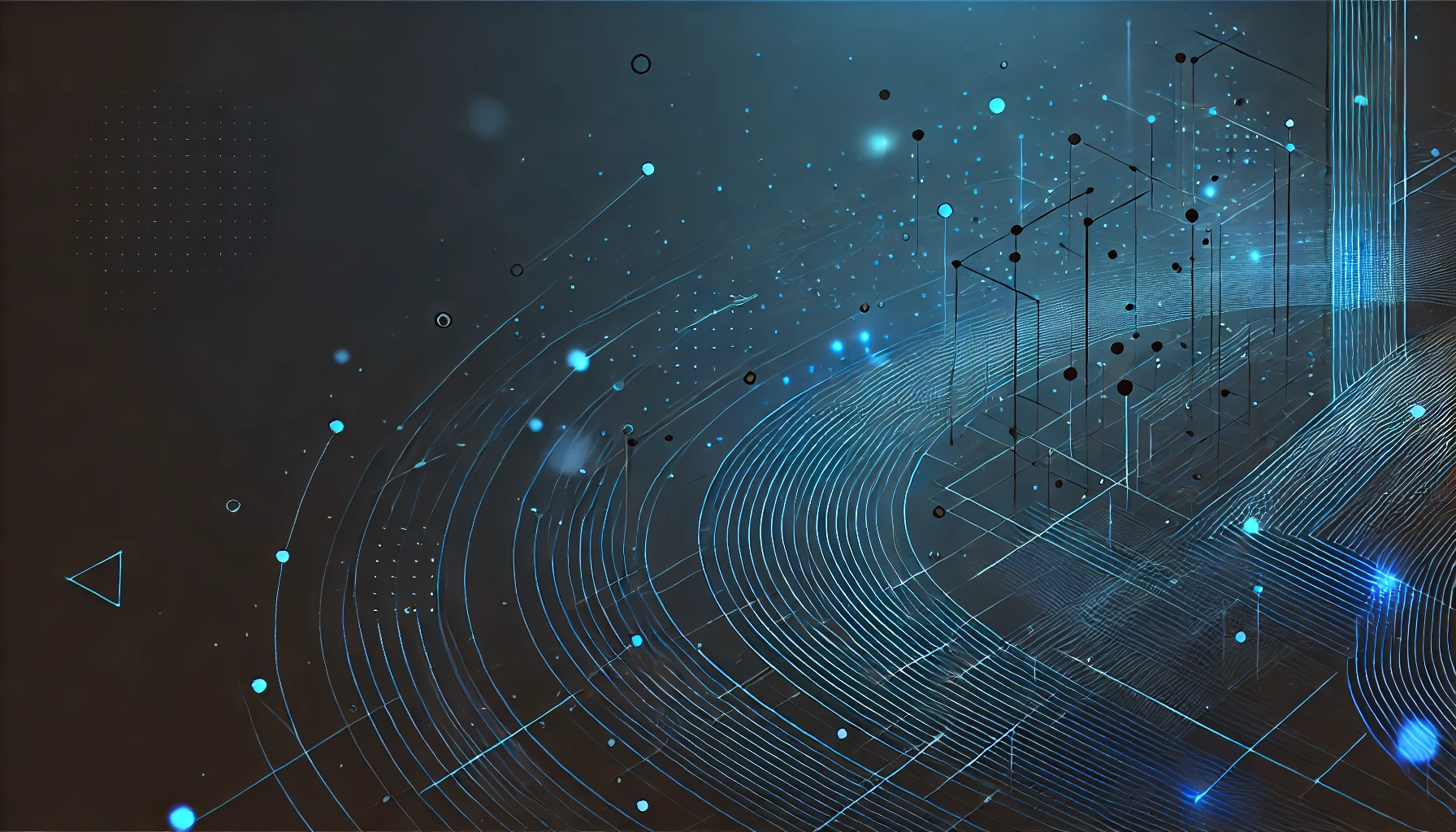
Don't ForgetCSS
Cheat Sheet
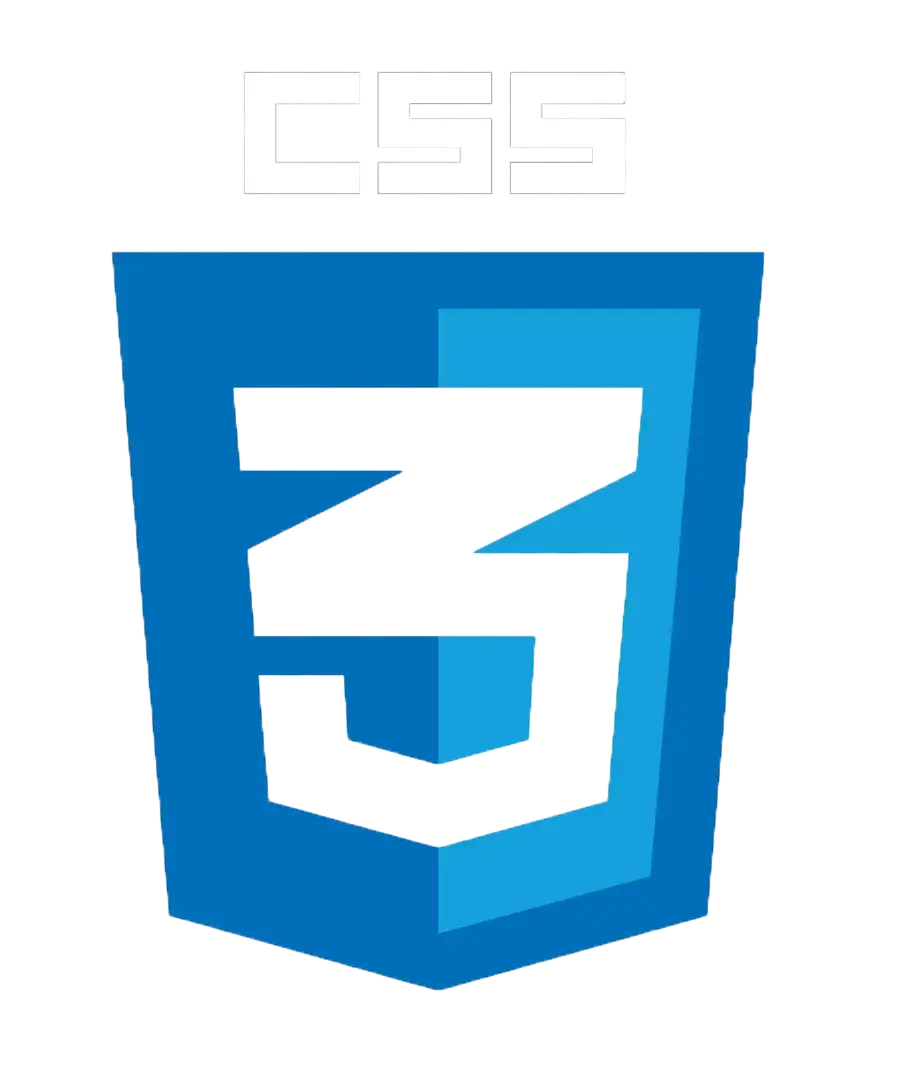
CSS Basics
Layout
CSS Tools & Extensions
Selectors
Target HTML elements
BASIC SELECTORS
/* Element selector */
p { color: blue; }
/* Class selector */
.highlight { background: yellow; }
/* ID selector */
#header { font-size: 24px; }
/* Universal selector */
* { margin: 0; padding: 0; }
/* Attribute selector */
input[type="text"] { border: 1px solid gray; }
/* Multiple selectors */
h1, h2, h3 { font-family: Arial; }
Basic ways to select elements in CSS
COMBINATORS
/* Descendant selector (space) */
.container p { margin: 10px; }
/* Child selector (>) */
.parent > .child { padding: 5px; }
/* Adjacent sibling (+) */
h2 + p { font-weight: bold; }
/* General sibling (~) */
h2 ~ p { color: gray; }
Combine selectors to target specific elements
ADVANCED SELECTORS
/* Pseudo-classes */
:link { color: blue; }
:visited { color: purple; }
:hover { background: yellow; }
:active { color: red; }
:focus { outline: 2px solid blue; }
/* Structural pseudo-classes */
:first-child { font-weight: bold; }
:last-child { margin-bottom: 0; }
:nth-child(2n) { background: gray; }
/* Pseudo-elements */
::before { content: '→'; }
::after { content: '←'; }
/* Attribute selectors */
[attr^='val'] { /* Starts with 'val' */ }
[attr$='ue'] { /* Ends with 'ue' */ }
[attr*='foo'] { /* Contains 'foo' */ }
[attr~='foo'] { /* Contains word 'foo' */ }
[attr|='en'] { /* Starts with 'en' followed by '-' */ }
Pseudo-classes target elements in specific states (like :hover for mouse-over). Pseudo-elements (::before, ::after) create virtual elements. Attribute selectors target elements based on their attribute values using pattern matching (^= start, $= end, *= contains).
SPECIFICITY
/* Specificity hierarchy (from highest to lowest) */
/* 1. !important */
.element { color: red !important; }
/* 2. Inline styles */
<div style="color: red;">...</div>
/* 3. IDs */
#header { color: red; } /* Specificity: 100 */
/* 4. Classes, attributes, pseudo-classes */
.nav { color: red; } /* Specificity: 010 */
[type="text"] { } /* Specificity: 010 */
:hover { } /* Specificity: 010 */
/* 5. Elements, pseudo-elements */
div { color: red; } /* Specificity: 001 */
::before { } /* Specificity: 001 */
/* Combined selectors add up */
div.nav[type="text"] { } /* Specificity: 021 */
CSS specificity determines which styles take precedence when multiple rules target the same element. The hierarchy from strongest to weakest is: !important > inline styles > ID selectors > classes/attributes > elements. Each type adds to the specificity score (e.g., class=10, element=1, so div.class=11).
Box Model
Layout and spacing
BOX MODEL BASICS
/* Box model properties */
.box {
/* Content dimensions */
width: 200px;
height: 100px;
/* Padding */
padding: 20px; /* All sides */
padding: 10px 20px; /* Vertical Horizontal */
padding: 10px 20px 15px; /* Top Horizontal Bottom */
padding: 5px 10px 15px 20px; /* Top Right Bottom Left */
/* Border */
border: 1px solid black;
border-radius: 5px;
/* Margin */
margin: 10px;
margin-top: 20px;
}
/* Box sizing */
* {
box-sizing: border-box; /* Include padding and border in width/height */
}
Control element spacing and borders
Images
Image handling
IMAGE PROPERTIES
/* Basic image styling */
.image {
object-fit: cover | contain | fill | none | scale-down;
object-position: center | top | 50% 50%;
aspect-ratio: 16 / 9;
}
/* Background images */
.element {
background-image: url('image.jpg');
background-size: cover | contain | 100% 100%;
background-position: center;
background-repeat: no-repeat;
/* Multiple backgrounds */
background:
url('overlay.png') center/cover no-repeat,
url('background.jpg') center/cover no-repeat;
}
/* Responsive images */
.image {
max-width: 100%;
height: auto;
}
/* Image sprites */
.icon {
background-image: url('sprites.png');
background-position: -20px -50px;
width: 20px;
height: 20px;
}
Style and handle images
Typography
Text styling and fonts
FONT PROPERTIES
/* Font basics */
.text {
font-family: Arial, sans-serif;
font-size: 16px;
font-weight: normal | bold | 700;
font-style: normal | italic | oblique;
font-variant: normal | small-caps;
}
/* Web fonts */
@font-face {
font-family: 'CustomFont';
src: url('font.woff2') format('woff2');
}
/* Google Fonts */
@import url('https://fonts.googleapis.com/css2?family=Roboto&display=swap');
Font family and basic properties
TEXT LAYOUT
/* Text layout */
.text {
text-align: left | right | center | justify;
text-indent: 2em;
line-height: 1.5;
letter-spacing: 1px;
word-spacing: 2px;
white-space: nowrap | pre | pre-wrap;
}
/* Text decoration */
.text {
text-decoration: none | underline | line-through;
text-transform: none | uppercase | lowercase | capitalize;
text-shadow: 2px 2px 2px rgba(0, 0, 0, 0.3);
}
/* Text overflow */
.text {
text-overflow: ellipsis;
overflow: hidden;
white-space: nowrap;
}
Text alignment and spacing
Layout Basics
Basic layout properties
DISPLAY PROPERTIES
/* Display types */
.element {
display: block; /* Stack vertically */
display: inline; /* Flow with text */
display: inline-block; /* Inline with block properties */
display: flex; /* Flexbox container */
display: grid; /* Grid container */
display: none; /* Hide element */
}
Control how elements are displayed
POSITIONING
/* Position types */
.element {
position: static; /* Default positioning */
position: relative; /* Relative to normal position */
position: absolute; /* Relative to positioned parent */
position: fixed; /* Relative to viewport */
position: sticky; /* Hybrid of relative/fixed */
/* Positioning coordinates */
top: 0;
right: 20px;
bottom: 0;
left: 20px;
z-index: 1; /* Stack order */
}
/* Float and Clear */
.element {
float: left | right | none;
clear: left | right | both | none;
}
/* Overflow handling */
.container {
overflow: visible | hidden | scroll | auto;
overflow-x: hidden;
overflow-y: auto;
}
Control element positioning and stacking
Flexbox
Flexible box layout
FLEX CONTAINER
/* Flex Container */
.container {
display: flex;
/* Main axis */
flex-direction: row | row-reverse | column | column-reverse;
justify-content: flex-start | flex-end | center | space-between | space-around;
/* Cross axis */
align-items: stretch | flex-start | flex-end | center | baseline;
align-content: flex-start | flex-end | center | space-between | space-around;
/* Wrapping */
flex-wrap: nowrap | wrap | wrap-reverse;
/* Shorthand */
flex-flow: row wrap; /* direction wrap */
}
Properties for flex containers
FLEX ITEMS
/* Flex Items */
.item {
/* Growth and shrink */
flex-grow: 0; /* Default 0 */
flex-shrink: 1; /* Default 1 */
flex-basis: auto; /* Default auto */
/* Shorthand */
flex: 0 1 auto; /* grow shrink basis */
flex: 1; /* Same as 1 1 0% */
/* Self alignment */
align-self: auto | flex-start | flex-end | center | baseline | stretch;
/* Order */
order: 0; /* Default 0, can be negative */
}
Properties for flex items
Grid
Grid layout system
GRID CONTAINER
/* Grid Container */
.container {
display: grid;
/* Define columns and rows */
grid-template-columns: 100px 100px 100px; /* Fixed width */
grid-template-columns: repeat(3, 1fr); /* Fractional units */
grid-template-columns: minmax(100px, 1fr); /* Responsive */
grid-template-rows: 100px auto;
/* Grid gaps */
gap: 20px; /* row-gap and column-gap */
/* Template areas */
grid-template-areas:
"header header"
"sidebar content";
}
Grid container setup
GRID ITEMS
/* Grid Items */
.item {
/* Grid lines */
grid-column: 1 / 3; /* start / end */
grid-row: 1 / 3;
/* Named grid areas */
grid-area: header; /* Must match template areas */
/* Auto placement */
grid-auto-flow: row | column | dense;
/* Alignment */
justify-self: start | end | center | stretch;
align-self: start | end | center | stretch;
}
Grid item positioning
Responsive Design
Adaptive layouts
MEDIA QUERIES
/* Basic media queries */
@media screen and (max-width: 768px) {
.element {
width: 100%;
}
}
/* Common breakpoints */
@media (max-width: 640px) { /* Mobile */ }
@media (min-width: 641px) and (max-width: 1024px) { /* Tablet */ }
@media (min-width: 1025px) { /* Desktop */ }
/* Device features */
@media (hover: hover) { /* Devices with hover */ }
@media (prefers-color-scheme: dark) { /* Dark mode */ }
Responsive breakpoints
RESPONSIVE UNITS
/* Relative units */
.element {
/* Font relative */
font-size: 1.5em; /* Relative to parent */
margin: 2rem; /* Relative to root */
/* Viewport relative */
width: 50vw; /* Viewport width */
height: 100vh; /* Viewport height */
padding: 5vmin; /* Viewport min */
/* Container queries */
container-type: inline-size;
@container (min-width: 400px) {
/* Styles */
}
}
Flexible units and containers
Preprocessors
CSS extensions
SASS/SCSS
// Variables
$primary-color: #333;
// Nesting
.container {
padding: 20px;
&__header {
color: $primary-color;
}
&:hover {
background: #eee;
}
}
// Mixins
@mixin flex-center {
display: flex;
justify-content: center;
align-items: center;
}
// Functions
@function calculate-width($n) {
@return $n * 100px;
}
// Use
.element {
@include flex-center;
width: calculate-width(2);
}
SASS preprocessor features
POSTCSS & MODERN CSS
/* CSS Modules */
.component {
composes: base from './base.css';
}
/* CSS Custom Properties */
:root {
--primary: #007bff;
--spacing: clamp(1rem, 2vw, 2rem);
}
/* Modern CSS Features */
.element {
/* Logical Properties */
margin-inline: auto;
padding-block: 1rem;
/* Color Functions */
color: rgb(from var(--primary) r g b / 0.8);
/* Container Queries */
container: inline-size;
}
Modern CSS tools and features
CSS VARIABLES & CALCULATIONS
/* CSS Custom Properties */
:root {
--primary-color: #007bff;
--spacing-unit: 8px;
--max-width: 1200px;
}
/* Using Variables */
.element {
color: var(--primary-color);
padding: var(--spacing-unit);
margin: var(--spacing-unit, 10px); /* Fallback value */
}
/* CSS Calculations */
.element {
width: calc(100% - 20px);
height: calc(100vh - var(--header-height));
font-size: calc(16px + 2vw);
}
CSS Custom Properties (variables) let you define reusable values with --name syntax. They can be changed with JavaScript, support fallback values, and inherit through the DOM. The calc() function performs mathematical operations, even mixing units (e.g., viewport units with pixels).
CSS Frameworks
Popular frameworks
UTILITY FRAMEWORKS
<!-- Tailwind CSS -->
<div class="flex items-center justify-between p-4 bg-white shadow-lg">
<h1 class="text-xl font-bold text-gray-800">Title</h1>
<button class="px-4 py-2 bg-blue-500 text-white rounded hover:bg-blue-600">
Button
</button>
</div>
<!-- Bootstrap Classes -->
<div class="d-flex align-items-center justify-content-between p-4 bg-white shadow">
<h1 class="h4 fw-bold text-dark">Title</h1>
<button class="btn btn-primary">Button</button>
</div>
Popular CSS frameworks