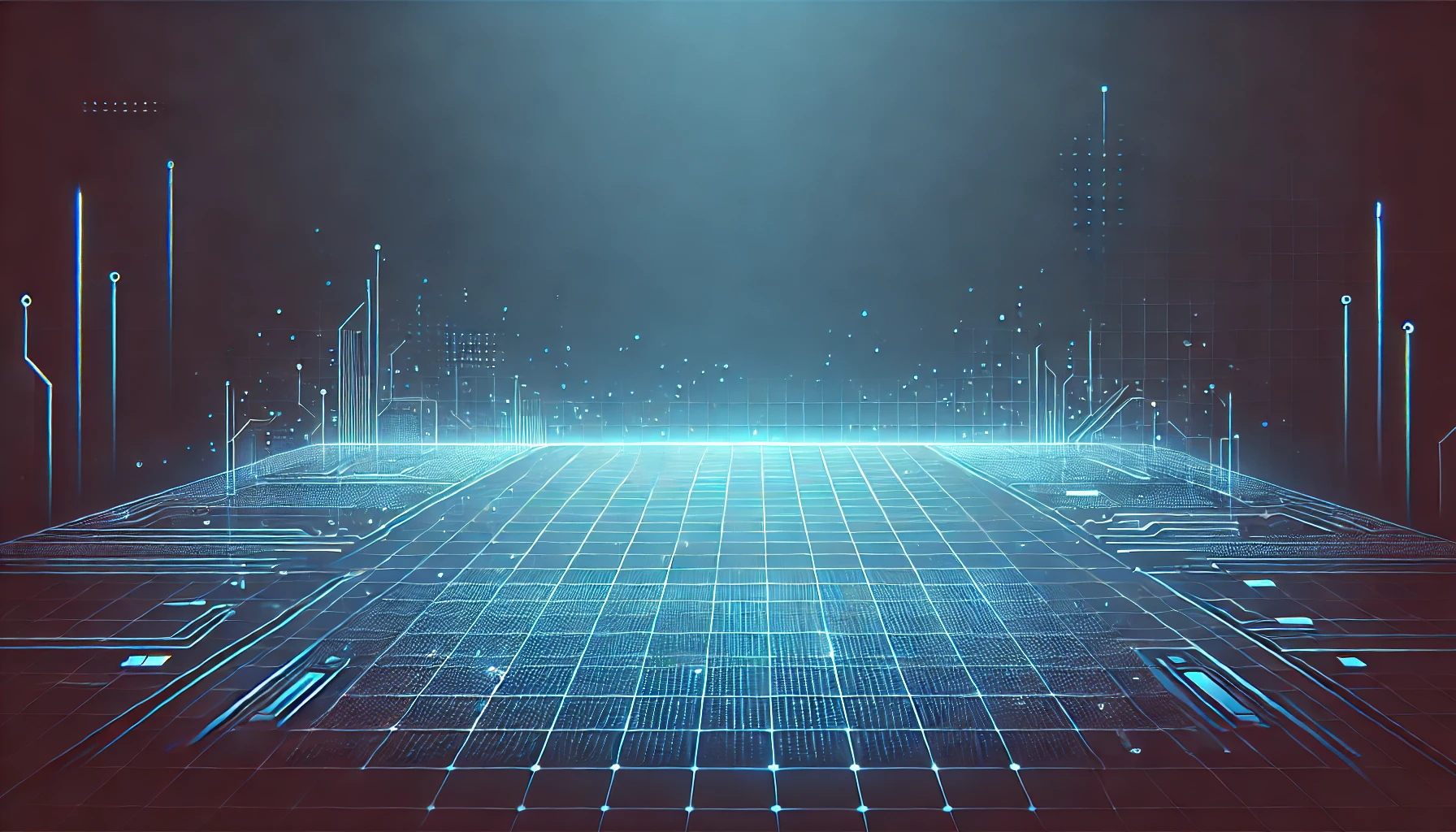
Don't ForgetPython
Cheat Sheet
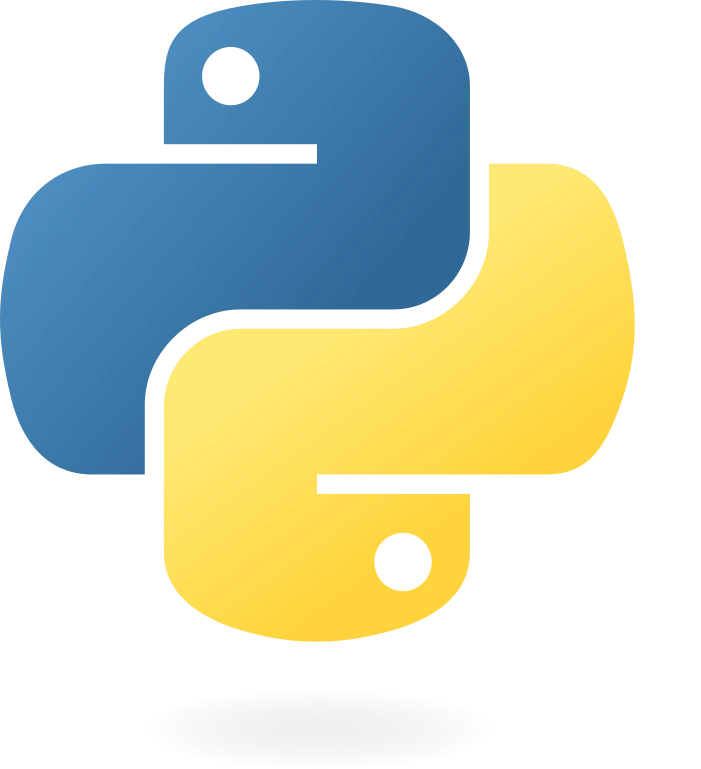
Python Basics
Advanced Python
Python Development
Web Development
Data Science, Machine Learning & AI
Variables & Types
Store and work with data
BASIC TYPES
# Numbers
age = 25 # Integer (whole number)
height = 1.75 # Float (decimal)
# Text
name = 'Alice' # String (text)
# True/False
is_student = True # Boolean
# Print variables
print(f'Name: {name}, Age: {age}')
# Check type
print(type(age)) # <class 'int'>
print(type(name)) # <class 'str'>
OPERATORS & TRUTHINESS
# Logical Operators
and # True if both operands are True
or # True if at least one operand is True
not # Inverts the boolean value
# Truthiness
bool('') # False (empty string)
bool([]) # False (empty list)
bool(0) # False
bool(None) # False
bool('text') # True (non-empty string)
bool([1, 2]) # True (non-empty list)
# Identity vs Equality
a = [1, 2, 3]
b = [1, 2, 3]
c = a
a == b # True (same value)
a is b # False (different objects)
a is c # True (same object)
# Operator Precedence
# 1. ()
# 2. **
# 3. *, /, //, %
# 4. +, -
# 5. <, <=, >, >=, ==, !=
# 6. not
# 7. and
# 8. or
COLLECTIONS
# List - Ordered collection []
fruits = ['apple', 'banana', 'orange']
fruits.append('grape') # Add item
fruits.remove('banana') # Remove item
# Dictionary - Key-value pairs {}
user = {
'name': 'Alice',
'age': 25
}
print(user['name']) # Get value
user['email'] = '[email protected]' # Add pair
# Tuple - Immutable list ()
point = (x, y) # Can't change after creation
PRIMITIVE TYPES & OPERATORS
# Numeric Types
x = 5 # int
y = 3.14 # float
z = 2 + 3j # complex
# Arithmetic Operators
x + y # Addition
x - y # Subtraction
x * y # Multiplication
x / y # Division (float)
x // y # Floor division
x % y # Modulus
x ** y # Exponentiation
# Comparison Operators
x == y # Equal to
x is y # Identity comparison
[] == [] # True
[] is [] # False (different objects)
# Operator Precedence
result = 2 + 3 * 4 # 14 (not 20)
result = (2 + 3) * 4 # 20
Control Flow
Making decisions in your code
IF STATEMENTS
# Basic if statement
age = 18
if age >= 18:
print('You can vote!')
else:
print('Too young to vote')
# elif for multiple conditions
grade = 85
if grade >= 90:
print('A')
elif grade >= 80:
print('B')
else:
print('C')
# One-line if (ternary)
status = 'adult' if age >= 18 else 'minor'
COMPARISONS
# Comparison operators
x == y # Equal to
x != y # Not equal to
x > y # Greater than
x < y # Less than
x >= y # Greater than or equal
x <= y # Less than or equal
# Multiple conditions
age = 25
income = 50000
if age > 18 and income >= 40000:
print('Loan approved')
# in operator
name = 'Alice'
if 'A' in name:
print('Name contains A')
ADVANCED CONTROL FLOW
# break and continue
for i in range(10):
if i == 3:
continue # Skip 3
if i == 8:
break # Stop at 8
print(i)
# try/except/else/finally
try:
result = x / y
except ZeroDivisionError:
print('Division by zero!')
else:
print('Division succeeded')
finally:
print('Cleanup code')
# Context Managers
class MyContext:
def __enter__(self):
print('Starting')
return self
def __exit__(self, exc_type, exc_val, exc_tb):
print('Cleaning up')
with MyContext():
print('Inside context')
ITERATORS & PROTOCOLS
# Iterator Protocol
class Counter:
def __init__(self, start, end):
self.start = start
self.end = end
def __iter__(self):
self.current = self.start
return self
def __next__(self):
if self.current > self.end:
raise StopIteration
result = self.current
self.current += 1
return result
# Using iterator
for num in Counter(1, 3):
print(num) # 1, 2, 3
# Iterator vs Iterable
iterable = [1, 2, 3] # Iterable
iterator = iter(iterable) # Iterator
print(next(iterator)) # 1
print(next(iterator)) # 2
Loops
Repeat actions in your code
FOR LOOPS
# Loop through a list
fruits = ['apple', 'banana', 'orange']
for fruit in fruits:
print(fruit)
# Loop with range
for i in range(5): # 0 to 4
print(i)
# Loop with index
for i, fruit in enumerate(fruits):
print(f'{i}: {fruit}')
# Loop through dictionary
user = {'name': 'John', 'age': 25}
for key, value in user.items():
print(f'{key}: {value}')
WHILE LOOPS
# Basic while loop
count = 0
while count < 5:
print(count)
count += 1
# Break and continue
while True:
text = input('Enter "quit" to exit: ')
if text == 'quit':
break # Exit the loop
if text == '':
continue # Skip to next iteration
print(text)
Functions
Reusable blocks of code
FUNCTION DECLARATION
def greet(name, greeting='Hello'):
"""Greet someone with a message.
Args:
name: Person's name
greeting: Optional greeting
Returns:
Formatted greeting string
"""
return f'{greeting}, {name}!'
'Hello, John!'
FLEXIBLE ARGUMENTS
# *args for variable positional args
def sum_all(*args):
return sum(args)
sum_all(1, 2, 3) # 6
# **kwargs for variable keyword args
def print_info(**kwargs):
for k, v in kwargs.items():
print(f'{k}: {v}')
ADVANCED FUNCTIONS
# Scope rules
global_var = 10
def outer():
outer_var = 20
def inner():
nonlocal outer_var # Access outer scope
global global_var # Access global scope
outer_var += 1
global_var += 1
return inner
# Closures
def counter():
count = 0
def increment():
nonlocal count
count += 1
return count
return increment
# Function attributes
def greet(name):
"""Greet someone."""
return f'Hello {name}!'
greet.short_desc = 'Greeting function'
print(greet.__doc__) # Access docstring
print(greet.__name__) # Function name
Strings
Working with text in Python
STRING BASICS
# String creation
name = 'Alice' # Single quotes
greeting = "Hello" # Double quotes
multiline = '''This is a
multiline string''' # Triple quotes
# String concatenation
full_name = 'John' + ' ' + 'Doe'
# String repetition
stars = '*' * 3 # '***'
# String length
len(name) # 5
# Access characters
first = name[0] # 'A'
last = name[-1] # 'e'
# Slicing
substr = name[1:3] # 'li'
reversed = name[::-1] # 'ecilA'
STRING METHODS
text = ' Hello, World! '
# Case methods
text.upper() # 'HELLO, WORLD!'
text.lower() # 'hello, world!'
text.title() # 'Hello, World!'
text.capitalize() # 'Hello, world!'
# Whitespace
text.strip() # 'Hello, World!'
text.lstrip() # 'Hello, World! '
text.rstrip() # ' Hello, World!'
# Search methods
text.find('World') # 8
text.index('World') # 8 (raises error if not found)
text.count('l') # 3
'World' in text # True
# Replace
text.replace('World', 'Python') # ' Hello, Python! '
STRING FORMATTING
name = 'Alice'
age = 25
# F-strings (Python 3.6+)
f'Name: {name}, Age: {age}' # 'Name: Alice, Age: 25'
f'{name=}, {age=}' # "name='Alice', age=25"
f'{3.14159:.2f}' # '3.14'
# Format method
'Name: {}, Age: {}'.format(name, age)
'Name: {n}, Age: {a}'.format(n=name, a=age)
# % operator (older style)
'Name: %s, Age: %d' % (name, age)
# Common format specifiers
f'{42:08d}' # '00000042' (padding)
f'{3.14159:8.2f}' # ' 3.14' (width.precision)
f'{42:<8d}' # '42 ' (left align)
f'{42:>8d}' # ' 42' (right align)
f'{42:^8d}' # ' 42 ' (center)
STRING OPERATIONS
# Splitting and joining
text = 'apple,banana,orange'
fruits = text.split(',') # ['apple', 'banana', 'orange']
words = 'Hello World'.split() # ['Hello', 'World']
# Join lists into string
','.join(['apple', 'banana']) # 'apple,banana'
' '.join(['Hello', 'World']) # 'Hello World'
# Check string properties
'hello123'.isalnum() # True (alphanumeric)
'hello'.isalpha() # True (alphabetic)
'123'.isdigit() # True (digits)
'Hello'.startswith('H') # True
'World'.endswith('d') # True
# Escape characters
print('Hello\nWorld') # Newline
print('Tab\there') # Tab
print('Path: C:\\Users') # Backslash
Lists
Working with Python lists
LIST BASICS
# Create lists
numbers = [1, 2, 3, 4, 5]
mixed = [1, 'hello', 3.14, True]
# Access elements
first = numbers[0] # First element
last = numbers[-1] # Last element
# Modify lists
numbers[0] = 10 # Change element
numbers.append(6) # Add to end
numbers.insert(0, 0) # Insert at index
numbers.extend([7, 8]) # Add multiple items
# Remove elements
numbers.remove(3) # Remove value
popped = numbers.pop() # Remove & return last
popped = numbers.pop(0) # Remove & return index
del numbers[1] # Delete at index
numbers.clear() # Remove all items
LIST METHODS
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3]
# Sorting
numbers.sort() # Sort in place
numbers.sort(reverse=True) # Sort descending
sorted_nums = sorted(numbers) # Return new sorted
# Finding elements
index = numbers.index(5) # First occurrence
count = numbers.count(1) # Count occurrences
# List operations
length = len(numbers) # List length
min_val = min(numbers) # Minimum value
max_val = max(numbers) # Maximum value
total = sum(numbers) # Sum of elements
# Check membership
if 5 in numbers: # Check if exists
print('Found 5!')
LIST OPERATIONS
# List comprehension
squares = [x**2 for x in range(5)]
evens = [x for x in range(10) if x % 2 == 0]
# Map, Filter, Reduce
numbers = [1, 2, 3, 4, 5]
# Map: Transform each element
doubled = list(map(lambda x: x*2, numbers))
# Same as: [x*2 for x in numbers]
# Filter: Select elements
evens = list(filter(lambda x: x%2==0, numbers))
# Same as: [x for x in numbers if x%2==0]
# Reduce: Combine elements
from functools import reduce
sum = reduce(lambda x,y: x+y, numbers)
# Same as: sum(numbers)
LIST SLICING
numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Basic slicing [start:end:step]
first_three = numbers[:3] # [0, 1, 2]
last_three = numbers[-3:] # [7, 8, 9]
middle = numbers[3:7] # [3, 4, 5, 6]
# With step
every_second = numbers[::2] # [0, 2, 4, 6, 8]
reversed = numbers[::-1] # [9, 8, 7, ..., 0]
# Modify slices
numbers[1:4] = [10, 20, 30] # Replace section
numbers[::2] = [0]*5 # Replace every second
# Copy lists
shallow_copy = numbers[:] # or numbers.copy()
deep_copy = copy.deepcopy(numbers)
ADVANCED COLLECTIONS
# Tuple Operations
tuple1 = (1, 2, 3)
tuple2 = ('a', 'b')
tuple3 = tuple1 + tuple2 # Concatenation
single_tuple = (42,) # Single-item tuple
# Set Operations
set1 = {1, 2, 3}
set2 = {3, 4, 5}
print(set1 | set2) # Union
print(set1 & set2) # Intersection
print(set1 - set2) # Difference
print(set1 ^ set2) # Symmetric difference
# Advanced Slicing
list1 = [0, 1, 2, 3, 4, 5]
print(list1[::2]) # Every second item
print(list1[::-1]) # Reverse
print(list1[1:4:2]) # Start:Stop:Step
# Mutability
# Mutable: list, dict, set
# Immutable: tuple, str, frozenset
# Collection Methods
list1.extend([6, 7]) # Add multiple items
list1.insert(0, -1) # Insert at index
dict1.update({'a': 1, 'b': 2}) # Update multiple
set1.update([4, 5]) # Add multiple items
Dictionaries
Key-value data structures
DICTIONARY BASICS
# Create dictionary
user = {
'name': 'Alice',
'age': 25,
'is_admin': False
}
# Access values
name = user['name'] # With key
age = user.get('age') # With get() method
role = user.get('role', 'user') # With default
# Modify dictionary
user['email'] = '[email protected]' # Add/update
user.update({'age': 26, 'city': 'NY'}) # Multiple
del user['is_admin'] # Remove key
popped = user.pop('age') # Remove and return
# Check keys
if 'name' in user:
print('Name exists')
DICTIONARY METHODS
# Dictionary views
keys = user.keys() # View of keys
values = user.values() # View of values
items = user.items() # View of pairs
# Loop through dictionary
for key in user:
print(key, user[key])
for key, value in user.items():
print(f'{key}: {value}')
# Dictionary comprehension
squares = {x: x**2 for x in range(5)}
# Merge dictionaries
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
merged = {**dict1, **dict2} # Python 3.5+
merged = dict1 | dict2 # Python 3.9+
NESTED DICTIONARIES
# Nested dictionary
users = {
'alice': {
'name': 'Alice Smith',
'age': 25,
'scores': [85, 92, 88]
},
'bob': {
'name': 'Bob Jones',
'age': 30,
'scores': [75, 80, 85]
}
}
# Access nested values
alice_age = users['alice']['age']
bob_scores = users['bob']['scores']
# Safely get nested values
from functools import reduce
def deep_get(dict_obj, path, default=None):
try:
return reduce(lambda d, k: d[k], path.split('.'), dict_obj)
except (KeyError, TypeError):
return default
# Usage
score = deep_get(users, 'alice.scores.0', 0)
Classes & Objects
Create your own data types
BASIC CLASS
# Define a class
class Dog:
# __init__ is called when creating a new instance
# self refers to the instance being created/used
# self is like 'this' in other languages - it's how an
# instance accesses its own attributes and methods
def __init__(self, name, age):
self.name = name # Instance attribute
self.age = age # Instance attribute
def bark(self):
# self must be the first parameter of instance methods
# so Python knows which instance to operate on
return f'{self.name} says woof!'
# Create and use objects
my_dog = Dog('Rex', 3) # Creates new instance, calls __init__
print(my_dog.name) # Access attribute via instance
print(my_dog.bark()) # Call method via instance
INHERITANCE
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Cat(Animal):
def speak(self):
return f'{self.name} says meow!'
class Dog(Animal):
def speak(self):
return f'{self.name} says woof!'
# Use different animals
animals = [Cat('Whiskers'), Dog('Rex')]
for animal in animals:
print(animal.speak())
SPECIAL METHODS
class Point:
# __init__: Constructor for new instances
def __init__(self, x, y):
self.x = x
self.y = y
# __str__: Human-readable string representation
def __str__(self):
return f'({self.x}, {self.y})'
# __repr__: Developer-readable representation
def __repr__(self):
return f'Point({self.x}, {self.y})'
# __add__: Defines + operator
def __add__(self, other):
return Point(self.x + other.x, self.y + other.y)
# __eq__: Defines == operator
def __eq__(self, other):
return self.x == other.x and self.y == other.y
# Usage
p1 = Point(1, 2)
p2 = Point(3, 4)
print(str(p1)) # Calls __str__
print(repr(p1)) # Calls __repr__
print(p1 + p2) # Calls __add__
print(p1 == p2) # Calls __eq__
PROPERTIES & DESCRIPTORS
class Circle:
def __init__(self, radius):
self._radius = radius # Protected attribute
@property # Getter
def radius(self):
return self._radius
@radius.setter # Setter
def radius(self, value):
if value < 0:
raise ValueError('Must be positive')
self._radius = value
@property # Computed property
def area(self):
return 3.14 * self._radius ** 2
# Usage
circle = Circle(5)
print(circle.radius) # Calls getter
circle.radius = 10 # Calls setter
print(circle.area) # Computes area
CLASS & STATIC METHODS
class Date:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
@classmethod # Alternative constructor
def from_string(cls, date_str):
year, month, day = map(int, date_str.split('-'))
return cls(year, month, day)
@staticmethod # Utility function
def is_valid_date(year, month, day):
return (1 <= month <= 12 and
1 <= day <= 31 and
year >= 0)
# Usage
date1 = Date(2024, 3, 21) # Regular constructor
date2 = Date.from_string('2024-3-21') # Class method
valid = Date.is_valid_date(2024, 3, 21) # Static method
INHERITANCE & MRO
class Animal:
def speak(self):
return 'Some sound'
class Flyable:
def fly(self):
return 'Flying!'
class Bird(Animal, Flyable): # Multiple inheritance
def speak(self):
return 'Chirp!'
# Method Resolution Order (MRO)
print(Bird.__mro__) # Shows inheritance chain
# Diamond problem
class A:
def method(self): return 'A'
class B(A):
def method(self): return 'B'
class C(A):
def method(self): return 'C'
class D(B, C): # Python's MRO resolves conflicts
pass
print(D().method()) # Calls B's method due to MRO
Error Handling
Handle exceptions and errors
TRY-EXCEPT
# Basic error handling
try:
x = 1 / 0
except ZeroDivisionError:
print('Cannot divide by zero!')
# Multiple exceptions
try:
num = int('abc')
except ValueError:
print('Invalid number')
except TypeError:
print('Type error')
# Catch all exceptions
try:
risky_operation()
except Exception as e:
print(f'Error: {e}')
finally:
print('Cleanup code')
CUSTOM EXCEPTIONS
# Define custom exception
class ValidationError(Exception):
def __init__(self, message):
self.message = message
super().__init__(self.message)
# Raise custom exception
def validate_age(age):
if age < 0:
raise ValidationError('Age cannot be negative')
if age > 150:
raise ValidationError('Invalid age')
# Using custom exception
try:
validate_age(-5)
except ValidationError as e:
print(e.message)
Working with Files
Read, write and manage files
BASIC FILE OPERATIONS
# Read entire file
with open('file.txt', 'r') as f:
content = f.read()
# Read line by line
with open('file.txt', 'r') as f:
for line in f:
print(line.strip())
# Write to file
with open('output.txt', 'w') as f:
f.write('Hello World!')
# Append to file
with open('log.txt', 'a') as f:
f.write('New log entry\n')
FILE MODES & ENCODING
# Common file modes
'r' # Read (default)
'w' # Write (overwrites)
'a' # Append
'r+' # Read and write
'b' # Binary mode
# Handle different encodings
with open('file.txt', 'r', encoding='utf-8') as f:
content = f.read()
# Binary files
with open('image.jpg', 'rb') as f:
data = f.read()
with open('copy.jpg', 'wb') as f:
f.write(data)
COMMON FILE FORMATS
# CSV files
import csv
# Read CSV
with open('data.csv', 'r') as f:
reader = csv.reader(f)
for row in reader:
print(row)
# Write CSV
with open('output.csv', 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(['name', 'age'])
writer.writerow(['Alice', 25])
# JSON files
import json
# Read JSON
with open('data.json', 'r') as f:
data = json.load(f)
# Write JSON
with open('output.json', 'w') as f:
json.dump(data, f, indent=2)
FILE SYSTEM OPERATIONS
import os
import shutil
# Check if file exists
os.path.exists('file.txt') # True/False
# Get file info
os.path.getsize('file.txt') # Size in bytes
os.path.getmtime('file.txt') # Modified time
# Create/remove directories
os.makedirs('new/folder') # Create nested dirs
os.rmdir('empty_folder') # Remove empty dir
shutil.rmtree('folder') # Remove dir and contents
# Copy/move files
shutil.copy('src.txt', 'dst.txt') # Copy file
shutil.move('old.txt', 'new.txt') # Move/rename
# List directory contents
os.listdir('.') # List files
for root, dirs, files in os.walk('.'): # Walk directory tree
print(f'Found {len(files)} files in {root}')
Modules & Packages
Organize and reuse code
MODULE BASICS
# mymodule.py
def greet(name):
return f'Hello, {name}!'
PI = 3.14159
# Using modules
import mymodule
print(mymodule.greet('Alice'))
# Import specific items
from mymodule import greet, PI
# Import with alias
import mymodule as mm
print(mm.greet('Bob'))
# Import all (not recommended)
from mymodule import *
PACKAGE STRUCTURE
# Directory structure
mypackage/
__init__.py
module1.py
module2.py
subpackage/
__init__.py
module3.py
# __init__.py
from .module1 import function1
from .module2 import function2
# Using packages
from mypackage import function1
from mypackage.subpackage import module3
ADVANCED MODULES
# Module search path
import sys
print(sys.path) # List of search paths
# __name__ == '__main__'
if __name__ == '__main__':
print('Running as script')
else:
print('Imported as module')
# Module attributes
print(__file__) # Module filename
print(__name__) # Module name
print(__package__) # Package name
# Package structure
mypackage/
__init__.py
module1.py
subpackage/
__init__.py
module2.py
# In __init__.py
from .module1 import * # Export module1 contents
__all__ = ['function1'] # Control what's imported
HTTP Requests
Make web requests with Python
BASIC REQUESTS
import requests
# GET request
response = requests.get('https://api.example.com/data')
print(response.status_code) # 200
print(response.json()) # Parse JSON response
# POST request with data
data = {'name': 'Alice', 'age': 25}
response = requests.post(
'https://api.example.com/users',
json=data
)
# Headers and params
headers = {'Authorization': 'Bearer token'}
params = {'page': 1, 'limit': 10}
response = requests.get(
'https://api.example.com/users',
headers=headers,
params=params
)
ADVANCED REQUESTS
# Handle errors
try:
response = requests.get('https://api.example.com')
response.raise_for_status() # Raise error for 4XX/5XX
data = response.json()
except requests.RequestException as e:
print(f'Error: {e}')
# Sessions for multiple requests
with requests.Session() as session:
session.headers.update({'Authorization': 'Bearer token'})
response1 = session.get('https://api.example.com/1')
response2 = session.get('https://api.example.com/2')
# File upload
files = {'file': open('image.jpg', 'rb')}
response = requests.post(
'https://api.example.com/upload',
files=files
)
JSON BASICS
import json
# Python dict to JSON string
data = {
'name': 'Alice',
'age': 25,
'cities': ['New York', 'London']
}
json_string = json.dumps(data, indent=2)
# JSON string to Python dict
data_dict = json.loads(json_string)
# Read/Write JSON files
with open('data.json', 'w') as f:
json.dump(data, f, indent=2)
with open('data.json', 'r') as f:
loaded_data = json.load(f)
Regular Expressions
Pattern matching in text
REGEX BASICS
import re
# Basic patterns
text = 'My email is [email protected]'
# Find all emails
emails = re.findall(r'\w+@\w+\.\w+', text)
# Common patterns:
'\d' # Any digit (0-9)
'\w' # Any word character (a-z, A-Z, 0-9, _)
'\s' # Any whitespace
'[aeiou]' # Any vowel
'^' # Start of string
'$' # End of string
'+' # One or more
'*' # Zero or more
'?' # Zero or one
COMMON EXAMPLES
# Match phone numbers
phone = '123-456-7890'
if re.match(r'\d{3}-\d{3}-\d{4}', phone):
print('Valid phone number')
# Replace text
text = 'My phone is 123-456-7890'
new_text = re.sub(r'\d{3}-\d{3}-\d{4}',
'XXX-XXX-XXXX',
text)
# Split string
text = 'apple,banana;orange:grape'
fruits = re.split(r'[,;:]', text)
# ['apple', 'banana', 'orange', 'grape']
Dates & Times
Working with dates and times
BASIC DATE OPERATIONS
from datetime import datetime, timedelta
# Current date and time
now = datetime.now()
print(now) # 2024-03-21 14:30:00
# Create specific date
date = datetime(2024, 3, 21)
# Format dates
formatted = now.strftime('%Y-%m-%d')
print(formatted) # '2024-03-21'
# Add/subtract time
tomorrow = now + timedelta(days=1)
last_week = now - timedelta(weeks=1)
PARSING DATES
from datetime import datetime
# Parse string to date
date_string = '2024-03-21'
date = datetime.strptime(date_string, '%Y-%m-%d')
# Common formats
'%Y-%m-%d' # '2024-03-21'
'%d/%m/%Y' # '21/03/2024'
'%B %d, %Y' # 'March 21, 2024'
'%H:%M:%S' # '14:30:00'
# Get parts of dates
print(now.year) # 2024
print(now.month) # 3
print(now.day) # 21
Generators
Create memory-efficient iterators
GENERATOR FUNCTION
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
# Use generator
fib = fibonacci()
for _ in range(5):
print(next(fib)) # 0, 1, 1, 2, 3
GENERATOR EXPRESSION
# List comprehension (stores all values)
numbers = [x**2 for x in range(1000000)]
# Generator expression (creates values as needed)
numbers = (x**2 for x in range(1000000))
# Memory efficient for large datasets
for num in numbers:
print(num) # Prints one at a time
ADVANCED GENERATORS
# Generator expressions
squares = (x**2 for x in range(1000000))
# Generator with send
def counter():
i = 0
while True:
val = yield i
if val is not None:
i = val
else:
i += 1
# Generator delegation
def gen1():
yield from range(3)
yield from 'abc'
# Memory efficiency
def read_large_file(file_path):
with open(file_path) as f:
while True:
line = f.readline()
if not line:
break
yield line
Decorators
Modify or enhance functions
BASIC DECORATOR
def timer(func):
def wrapper(*args, **kwargs):
import time
start = time.time()
result = func(*args, **kwargs)
print(f'Time: {time.time() - start}')
return result
return wrapper
@timer
def slow_function():
time.sleep(1)
return 'Done!'
DECORATOR WITH ARGS
def repeat(times):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(times=3)
def greet(name):
print(f'Hello {name}')
Lambda Functions
Write quick, anonymous functions
LAMBDA BASICS
# Basic lambda function
square = lambda x: x**2
print(square(5)) # 25
# With multiple arguments
sum = lambda a, b: a + b
print(sum(3, 4)) # 7
# In sorting
names = ['Alice', 'Bob', 'Charlie']
names.sort(key=lambda x: len(x))
# With map and filter
numbers = [1, 2, 3, 4, 5]
doubled = list(map(lambda x: x*2, numbers))
evens = list(filter(lambda x: x%2==0, numbers))
FUNCTIONAL PROGRAMMING
from functools import reduce
# Map: Transform items
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
# Filter: Select items
evens = list(filter(lambda x: x%2==0, numbers))
# Reduce: Combine items
sum = reduce(lambda x,y: x+y, numbers)
# List comprehension alternatives
squares = [x**2 for x in numbers]
evens = [x for x in numbers if x%2==0]
Context Managers
Manage resources with 'with' statements
WITH STATEMENT
# Basic file handling
with open('file.txt', 'r') as file:
content = file.read()
# File automatically closes
# Custom context manager
from contextlib import contextmanager
@contextmanager
def timer():
start = time.time()
yield
print(f'Time: {time.time() - start}')
with timer():
time.sleep(1)
Python Setup
Get started with Python development
INSTALLATION
# Windows
# 1. Download from python.org
# 2. Run installer (check 'Add Python to PATH')
# 3. Verify in terminal:
python --version
# macOS
# Using Homebrew:
brew install python
# Linux (Ubuntu/Debian)
sudo apt update
sudo apt install python3
# Verify pip installation
pip --version
# Update pip
python -m pip install --upgrade pip
IDE SETUP
# Popular Python IDEs:
# VS Code
# 1. Install VS Code
# 2. Install Python extension
# 3. Install Python Language Server
# PyCharm
# 1. Download from jetbrains.com
# 2. Install and set up interpreter
# Sublime Text
# 1. Install Package Control
# 2. Install 'Anaconda' package
# Recommended VS Code extensions:
# - Python (Microsoft)
# - Pylance
# - Python Docstring Generator
# - Python Test Explorer
Python Versions
Manage different Python versions
VERSION MANAGEMENT
# Check Python version
python --version
python3 --version
# Using pyenv (version manager)
# Install pyenv:
brew install pyenv # macOS
# List available versions
pyenv install --list
# Install specific version
pyenv install 3.9.7
# Set global version
pyenv global 3.9.7
# Set local version (per project)
pyenv local 3.8.12
# View installed versions
pyenv versions
VERSION COMPATIBILITY
# Python 2 vs 3 differences
# Print statement
print 'Hello' # Python 2
print('Hello') # Python 3
# Integer division
3 / 2 # Python 2: 1
3 / 2 # Python 3: 1.5
# Unicode strings
u'hello' # Python 2
'hello' # Python 3 (all strings are Unicode)
# Input function
raw_input() # Python 2
input() # Python 3
Virtual Environments
Isolate project dependencies
VENV BASICS
# Create virtual environment
python -m venv myenv
# Activate virtual environment
# Windows:
myenv\Scripts\activate
# macOS/Linux:
source myenv/bin/activate
# Deactivate
deactivate
# Install packages in venv
pip install requests
# Generate requirements.txt
pip freeze > requirements.txt
# Install from requirements.txt
pip install -r requirements.txt
VIRTUALENV TOOLS
# Poetry - Modern dependency management
# Install:
curl -sSL https://install.python-poetry.org | python3 -
# Create new project
poetry new myproject
# Add dependencies
poetry add requests
# Conda - Scientific Python distribution
# Create environment
conda create -n myenv python=3.9
# Activate conda environment
conda activate myenv
# Install packages
conda install numpy pandas
Debugging
Find and fix problems in your code
PDB DEBUGGING
# Start pdb
import pdb
def complex_function(x):
y = x * 2
pdb.set_trace() # Breakpoint
z = y + 3
return z
# Common pdb commands:
n # Next line
s # Step into function
c # Continue execution
q # Quit debugger
p var # Print variable
w # Show call stack
l # List source code
ll # List more source
b 10 # Set breakpoint at line 10
clear 10 # Clear breakpoint
h # Help
IPDB ENHANCED DEBUGGER
# Install ipdb
# pip install ipdb
import ipdb
def process_data(data):
result = []
ipdb.set_trace() # Better interface than pdb
for item in data:
# Process items...
result.append(item * 2)
return result
# Features over pdb:
# - Syntax highlighting
# - Tab completion
# - Better variable inspection
# - Command history
Jupyter Notebooks
Interactive Python development
JUPYTER BASICS
# Install Jupyter
# pip install jupyter
# Start Jupyter
# jupyter notebook
# Basic commands in notebook:
# Shift + Enter Run cell
# Ctrl + Enter Run cell in place
# Alt + Enter Run and add cell
# Esc Command mode
# A Add cell above
# B Add cell below
# DD Delete cell
# M Change to Markdown
# Y Change to Python
MAGIC COMMANDS
%matplotlib inline # Show plots in notebook
%time # Time execution
%%time # Time cell execution
%who # List variables
%load_ext autoreload # Auto-reload modules
%autoreload 2 # Reload all modules
# HTML display
from IPython.display import HTML
HTML('<h1>Hello</h1>')
# Rich output
import pandas as pd
df = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
df # Displays as nice table
Package Management
Manage Python packages and dependencies
PIP BASICS
# Install package
pip install requests
# Install specific version
pip install requests==2.26.0
# Upgrade package
pip install --upgrade requests
# Uninstall package
pip uninstall requests
# List installed packages
pip list
# Show package info
pip show requests
DEPENDENCY MANAGEMENT
# Create requirements.txt
pip freeze > requirements.txt
# Install from requirements.txt
pip install -r requirements.txt
# Using setup.py
from setuptools import setup
setup(
name='mypackage',
version='0.1',
packages=['mypackage'],
install_requires=[
'requests>=2.25.1',
'pandas>=1.2.0'
]
)
Web Frameworks
Choose the right framework for your project
DJANGO
# Django - The batteries-included framework
Pros:
- Full-featured admin interface
- Built-in security features
- Great for large projects
- Excellent documentation
- ORM for database handling
Cons:
- Steeper learning curve
- Can be overkill for small projects
- Less flexible than Flask
Best for:
- Large enterprise projects
- Complex database operations
- Teams with varying experience
FLASK
# Flask - The lightweight framework
Pros:
- Easy to learn and use
- Highly flexible
- Perfect for small projects
- Great for APIs
- Minimal boilerplate
Cons:
- Need to add features manually
- Less structured than Django
- Can become complex as project grows
Best for:
- Small to medium projects
- Microservices
- Learning web development
FASTAPI
# FastAPI - The modern, fast framework
Pros:
- Very high performance
- Automatic API documentation
- Modern Python features
- Great type hints support
- Easy async support
Cons:
- Newer, smaller community
- Less full-stack features
- Fewer tutorials available
Best for:
- Modern API development
- High-performance needs
- Type-safe applications
REST APIs
Build web APIs with Python
FLASK API
from flask import Flask, request, jsonify
app = Flask(__name__)
# Basic GET endpoint
@app.route('/api/users', methods=['GET'])
def get_users():
users = [
{'id': 1, 'name': 'Alice'},
{'id': 2, 'name': 'Bob'}
]
return jsonify(users)
# POST endpoint with data
@app.route('/api/users', methods=['POST'])
def create_user():
data = request.get_json()
# Add user to database...
return jsonify({
'message': 'User created',
'user': data
}), 201
# Dynamic routes
@app.route('/api/users/<int:user_id>')
def get_user(user_id):
# Get user from database...
return jsonify({'id': user_id, 'name': 'Alice'})
FASTAPI EXAMPLE
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
# Data model
class User(BaseModel):
name: str
email: str
age: int
# GET with path parameters
@app.get('/users/{user_id}')
async def get_user(user_id: int):
# Get user from database...
if not user:
raise HTTPException(status_code=404)
return {'id': user_id, 'name': 'Alice'}
# POST with request body
@app.post('/users')
async def create_user(user: User):
# Validate automatically!
return {
'message': 'Created',
'user': user.dict()
}
Authentication
Secure your web applications
JWT AUTHENTICATION
from flask import Flask, jsonify, request
import jwt
from functools import wraps
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
def token_required(f):
@wraps(f)
def decorated(*args, **kwargs):
token = request.headers.get('Authorization')
if not token:
return jsonify({'message': 'Token missing'}), 401
try:
data = jwt.decode(
token,
app.config['SECRET_KEY'],
algorithms=['HS256']
)
except:
return jsonify({'message': 'Invalid token'}), 401
return f(*args, **kwargs)
return decorated
@app.route('/login')
def login():
# Verify credentials...
token = jwt.encode(
{'user_id': 123},
app.config['SECRET_KEY'],
algorithm='HS256'
)
return jsonify({'token': token})
SESSION AUTHENTICATION
from flask import Flask, session, redirect
from functools import wraps
app = Flask(__name__)
app.secret_key = 'your-secret-key'
def login_required(f):
@wraps(f)
def decorated(*args, **kwargs):
if 'user_id' not in session:
return redirect('/login')
return f(*args, **kwargs)
return decorated
@app.route('/login', methods=['POST'])
def login():
# Verify credentials...
session['user_id'] = 123
return redirect('/dashboard')
@app.route('/logout')
def logout():
session.pop('user_id', None)
return redirect('/login')
Database Integration
Connect to databases in Python
SQLALCHEMY ORM
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Setup
engine = create_engine('sqlite:///app.db')
Base = declarative_base()
Session = sessionmaker(bind=engine)
# Define models
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String, unique=True)
# Create tables
Base.metadata.create_all(engine)
# Use the database
session = Session()
# Create
user = User(name='Alice', email='[email protected]')
session.add(user)
session.commit()
# Query
users = session.query(User).filter_by(name='Alice').all()
for user in users:
print(user.name)
MONGODB WITH PYMONGO
from pymongo import MongoClient
# Connect to MongoDB
client = MongoClient('mongodb://localhost:27017/')
db = client['mydatabase']
# Create
user = {
'name': 'Alice',
'email': '[email protected]',
'age': 25
}
result = db.users.insert_one(user)
# Query
user = db.users.find_one({'name': 'Alice'})
# Update
db.users.update_one(
{'name': 'Alice'},
{'$set': {'age': 26}}
)
# Delete
db.users.delete_one({'name': 'Alice'})
# Query with filters
users = db.users.find(
{'age': {'$gt': 20}}
).sort('name')
Templates & Frontend
Create dynamic web pages
JINJA TEMPLATES
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/users')
def users():
users = [
{'name': 'Alice', 'age': 25},
{'name': 'Bob', 'age': 30}
]
return render_template(
'users.html',
users=users
)
# users.html
'''
<!DOCTYPE html>
<html>
<body>
{% for user in users %}
<div class="user">
<h2>{{ user.name }}</h2>
<p>Age: {{ user.age }}</p>
</div>
{% endfor %}
</body>
</html>
'''
HANDLING FORMS
from flask import Flask, request, render_template
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField
from wtforms.validators import DataRequired
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
class LoginForm(FlaskForm):
username = StringField('Username',
validators=[DataRequired()])
password = PasswordField('Password',
validators=[DataRequired()])
@app.route('/login', methods=['GET', 'POST'])
def login():
form = LoginForm()
if form.validate_on_submit():
# Process login...
return redirect('/dashboard')
return render_template('login.html', form=form)
# login.html
'''
<form method="POST">
{{ form.csrf_token }}
<div>
{{ form.username.label }}
{{ form.username }}
</div>
<div>
{{ form.password.label }}
{{ form.password }}
</div>
<button type="submit">Login</button>
</form>
'''
Data Libraries
Essential tools for data analysis
PANDAS
# Pandas - Data manipulation and analysis
import pandas as pd
# Read data
df = pd.read_csv('data.csv')
# Basic operations
df.head() # First 5 rows
df.describe() # Statistical summary
df['age'].mean() # Average age
# Data cleaning
df.dropna() # Remove missing values
df.fillna(0) # Fill missing with zero
# Grouping & aggregation
df.groupby('city')['salary'].mean()
NUMPY
# NumPy - Numerical computing
import numpy as np
# Create arrays
arr = np.array([1, 2, 3])
zeros = np.zeros(5)
random = np.random.rand(3, 3)
# Math operations
print(arr * 2) # Element-wise multiplication
print(arr.mean()) # Average
print(arr.max()) # Maximum value
# Linear algebra
a = np.array([[1, 2], [3, 4]])
b = np.array([[5, 6], [7, 8]])
np.dot(a, b) # Matrix multiplication
VISUALIZATION
# Matplotlib vs Seaborn vs Plotly
# Matplotlib - Complete control
Pros:
- Full control over plots
- Industry standard
- Works everywhere
Cons:
- Verbose code
- Less attractive defaults
# Seaborn - Statistical plots
Pros:
- Beautiful defaults
- Statistical plots built-in
- Works with pandas
Cons:
- Less flexible
- Limited to static plots
# Plotly - Interactive plots
Pros:
- Interactive plots
- Web-ready
- Modern look
Cons:
- Larger file sizes
- Can be slower
Machine Learning Basics
Get started with AI and ML
DATA HANDLING
# NumPy - Work with numbers
import numpy as np
# Create an array
numbers = np.array([1, 2, 3, 4, 5])
# Basic operations
print(numbers * 2) # Multiply all by 2
print(numbers.mean()) # Average
# Pandas - Work with tables
import pandas as pd
# Create a simple dataset
data = {
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'city': ['NY', 'SF', 'LA']
}
df = pd.DataFrame(data)
# Basic analysis
print(df.head()) # View data
print(df['age'].mean()) # Average age
SIMPLE ML MODEL
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Prepare data
X = [[1], [2], [3], [4], [5]] # Features
y = [2, 4, 6, 8, 10] # Target
# Split into training and testing
X_train, X_test, y_train, y_test = \
train_test_split(X, y, test_size=0.2)
# Create and train model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
VISUALIZE DATA
import matplotlib.pyplot as plt
import seaborn as sns
# Basic line plot
plt.plot([1, 2, 3, 4], [1, 4, 2, 3])
plt.title('Simple Line Plot')
plt.show()
# Scatter plot with Seaborn
sns.scatterplot(data=df, x='age', y='salary')
plt.title('Age vs Salary')
plt.show()
# Simple bar chart
sns.barplot(data=df, x='city', y='age')
plt.title('Average Age by City')
plt.show()
Deep Learning
Neural networks and deep learning
TENSORFLOW
import tensorflow as tf
# Create a simple neural network
model = tf.keras.Sequential([
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile model
model.compile(
optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy']
)
# Train model
model.fit(x_train, y_train, epochs=5)
# Make predictions
predictions = model.predict(x_test)
PYTORCH
import torch
import torch.nn as nn
# Define neural network
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Create model
model = Net()
# Define loss and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters())
# Training loop
for epoch in range(5):
for data, target in train_loader:
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
Data Preprocessing
Clean and prepare data for analysis
DATA CLEANING
import pandas as pd
import numpy as np
from sklearn.preprocessing import StandardScaler
# Handle missing values
df.dropna() # Remove rows with missing values
df.fillna(df.mean()) # Fill with mean
df.interpolate() # Interpolate values
# Remove duplicates
df.drop_duplicates()
# Handle outliers
z_scores = np.abs(stats.zscore(df))
df_no_outliers = df[(z_scores < 3).all(axis=1)]
# Scale features
scaler = StandardScaler()
df_scaled = scaler.fit_transform(df)
FEATURE ENGINEERING
# One-hot encoding
pd.get_dummies(df['category'])
# Binning
pd.cut(df['age'], bins=5)
# Custom features
def create_features(df):
df['age_squared'] = df['age'] ** 2
df['price_per_sqft'] = df['price'] / df['sqft']
return df
# Date features
df['year'] = df['date'].dt.year
df['month'] = df['date'].dt.month
df['day_of_week'] = df['date'].dt.dayofweek
Model Evaluation
Evaluate and improve models
EVALUATION METRICS
from sklearn.metrics import (
accuracy_score, precision_score,
recall_score, f1_score,
confusion_matrix, roc_auc_score
)
# Classification metrics
accuracy = accuracy_score(y_true, y_pred)
precision = precision_score(y_true, y_pred)
recall = recall_score(y_true, y_pred)
f1 = f1_score(y_true, y_pred)
# Regression metrics
from sklearn.metrics import (
mean_squared_error,
mean_absolute_error,
r2_score
)
mse = mean_squared_error(y_true, y_pred)
mae = mean_absolute_error(y_true, y_pred)
r2 = r2_score(y_true, y_pred)
CROSS VALIDATION
from sklearn.model_selection import (
cross_val_score,
KFold,
GridSearchCV
)
# Basic cross-validation
scores = cross_val_score(model, X, y, cv=5)
# K-Fold cross-validation
kf = KFold(n_splits=5, shuffle=True)
for train_idx, test_idx in kf.split(X):
X_train, X_test = X[train_idx], X[test_idx]
y_train, y_test = y[train_idx], y[test_idx]
# Grid search with cross-validation
params = {'C': [0.1, 1, 10], 'kernel': ['rbf', 'linear']}
grid = GridSearchCV(model, params, cv=5)
grid.fit(X, y)
print(grid.best_params_)
Computer Vision
Image processing and analysis
OPENCV BASICS
import cv2
import numpy as np
# Read and display image
img = cv2.imread('image.jpg')
cv2.imshow('Image', img)
cv2.waitKey(0)
# Basic operations
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(img, (5,5), 0)
edges = cv2.Canny(gray, 100, 200)
# Object detection
face_cascade = cv2.CascadeClassifier(
cv2.data.haarcascades + 'haarcascade_frontalface_default.xml'
)
faces = face_cascade.detectMultiScale(gray, 1.3, 5)
IMAGE CLASSIFICATION
import tensorflow as tf
from tensorflow.keras.applications import ResNet50
from tensorflow.keras.preprocessing import image
# Load pre-trained model
model = ResNet50(weights='imagenet')
# Prepare image
img = image.load_img('cat.jpg', target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = tf.keras.applications.resnet50.preprocess_input(x)
# Predict
predictions = model.predict(x)
decoded = tf.keras.applications.resnet50.decode_predictions(predictions)
Natural Language Processing
Process and analyze text data
NLTK BASICS
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
# Tokenization
text = "Natural language processing is fascinating!"
tokens = word_tokenize(text)
# Remove stopwords
stop_words = set(stopwords.words('english'))
filtered = [w for w in tokens if w not in stop_words]
# Lemmatization
lemmatizer = WordNetLemmatizer()
lemmas = [lemmatizer.lemmatize(w) for w in filtered]
# Part of speech tagging
pos_tags = nltk.pos_tag(tokens)
TRANSFORMERS
from transformers import pipeline
# Sentiment analysis
sentiment = pipeline('sentiment-analysis')
result = sentiment('I love this product!')
# Text generation
generator = pipeline('text-generation')
text = generator('Once upon a time', max_length=50)
# Question answering
qa = pipeline('question-answering')
context = 'Paris is the capital of France.'
question = 'What is the capital of France?'
answer = qa(question=question, context=context)