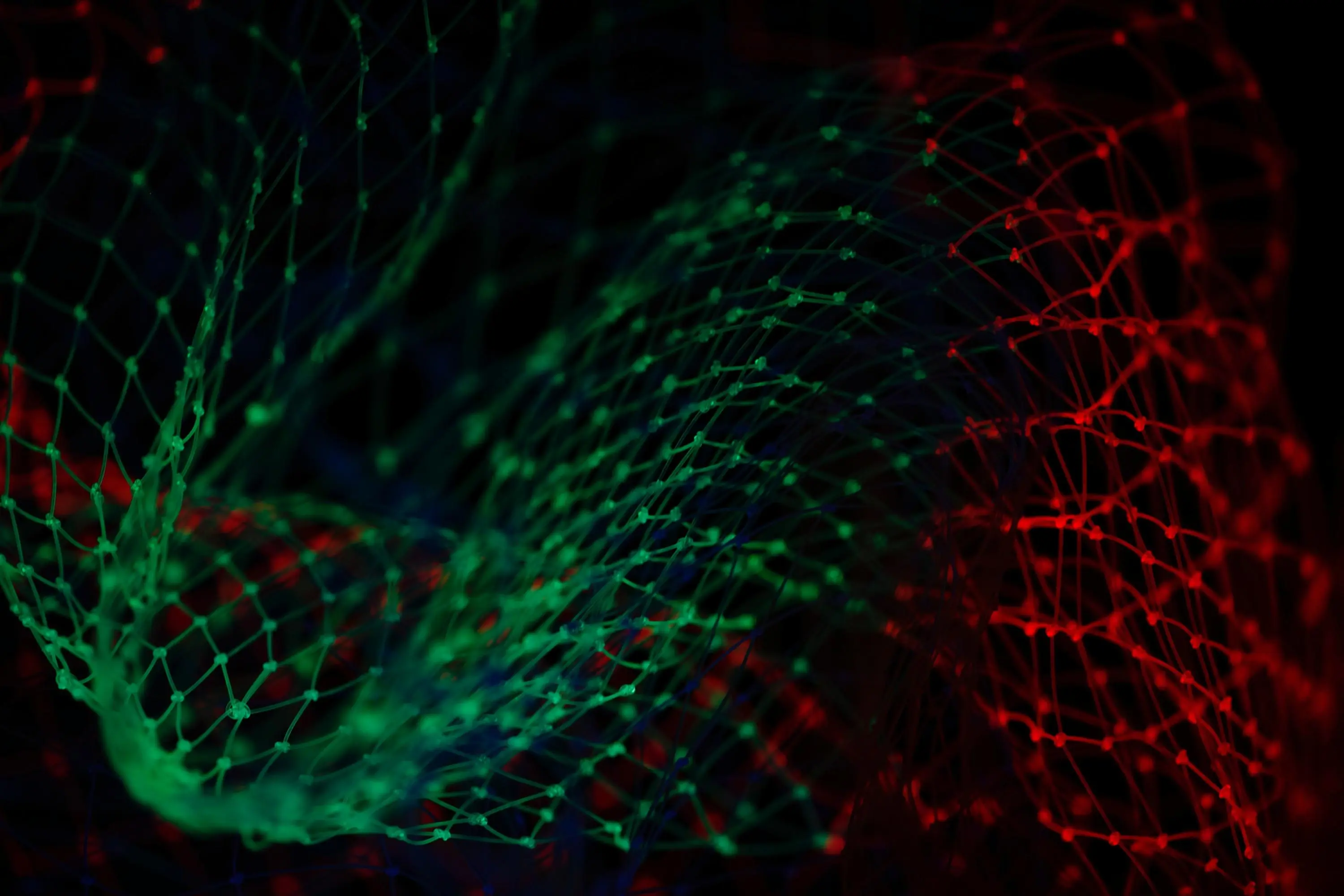
Don't ForgetJavaScript
Cheat Sheet
JavaScript Fundamentals
JavaScript-Specific
Additional Topics
Why JavaScript?
Why JavaScript dominates modern development
MOST POPULAR LANGUAGE
// Stack Overflow 2023 Survey
JavaScript: 63.6%
HTML/CSS: 52.0%
Python: 49.2%
SQL: 47.7%
TypeScript: 40.5%
WHERE IT RUNS
// Frontend (browsers)
websites, web apps
// Backend (Node.js)
servers, APIs
// Mobile (React Native)
iOS, Android apps
// Desktop (Electron)
Windows, Mac, Linux apps
JOB OPPORTUNITIES
// Average US Salaries (2024)
Junior Dev: $76,526
Mid-Level: $108,431
Senior: $147,192
// Job Openings
178,000+ JavaScript jobs
on LinkedIn USA
MASSIVE ECOSYSTEM
// NPM Package Registry
Total Packages: 2.1M+
Weekly Downloads: 25B+
Active Users: 17M+
EASY TO START
// To run JavaScript you need:
1. A web browser
2. A text editor
// That's it! No complex
// setup required
Variables & Types
The building blocks of JavaScript programs
DECLARING VARIABLES
// let - can be changed later
let score = 0
score = 10
// const - can't be changed
const name = 'John'
// name = 'Jane' // Error!
// var - avoid (outdated)
DATA TYPES
typeof "hello" // text (string)
typeof 42 // number
typeof true // true/false (boolean)
typeof {} // object
typeof [] // array (special object)
CHANGING TYPES
Number("42") // turns "42" into 42
String(42) // turns 42 into "42"
Boolean(1) // turns 1 into true
Functions
Reusable blocks of code
FUNCTION DECLARATION
function greet(name) {
return `Hello ${name}!`
}
greet('John') // call the function
"Hello John!"
FUNCTION EXPRESSION
const greet = function(name) {
return `Hello ${name}!`
}
greet('John')
"Hello John!"
PARAMETERS & DEFAULTS
function greet(name = 'friend') {
return `Hello ${name}!`
}
greet() // uses default
greet('John') // uses 'John'
"Hello friend!"
"Hello John!"
RETURN VALUES
function add(a, b) {
return a + b
// code after return is never run
}
const sum = add(2, 3) // returns 5
5
Loops & Iteration
Different ways to repeat code
FOR LOOP
// counts from 0 to 2
for (let count = 0; count < 3; count++) {
console.log(count)
}
0
1
2
FOR...OF LOOP
const fruits = ['apple', 'banana', 'orange']
for (let fruit of fruits) {
console.log(fruit)
}
apple
banana
orange
WHILE LOOP
let count = 0
while (count < 3) {
console.log(count)
count = count + 1
}
0
1
2
Conditionals
Make decisions in your code
IF...ELSE
if (age >= 18) {
console.log('Adult')
} else if (age >= 13) {
console.log('Teen')
} else {
console.log('Child')
}
TERNARY OPERATOR
const status = age >= 18 ? 'Adult' : 'Minor'
SWITCH
switch (fruit) {
case 'apple':
console.log('Selected apple')
break
default:
console.log('No fruit selected')
}
String & Array
These methods create copies of the original (except for splice)
SLICE
[1, 2, 3].slice(0, 1)
[1]
SPLIT
"Jun-1".split("-")
["Jun", "1"]
JOIN
["Jun", "1"].join("-")
"Jun-1"
SPLICE
[4, 2, 3].splice(0, 1)
[4]
original array is set to [2, 3]
MAP
[1, 2, 3].map(num => num * 2)
[2, 4, 6]
FILTER
[1, 2, 3].filter(num => num > 1)
[2, 3]
REDUCE
[1, 2, 3].reduce((sum, num) =>
sum + num, 0)
6
Arrow Functions
Replace the function keyword with => after your arguments and you have an arrow function
ARROW FUNCTION DECLARATION
const add = (num1, num2) => {
return num1 + num2
}
AUTO RETURNS
(num1, num2) => num1 + num2
AUTO RETURNS II
(num1, num2) => (
num1 + num2
)
SINGLE PARAMETER
word => word.toUpperCase()
Objects
Powerful, quick storage and retrieval
KEY LITERALS
obj.a OR obj["a"]
KEY WITH VARIABLE
obj[a]
FOR IN... LOOPS
for (let key in obj) ...
OBJECT.KEYS
Object.keys({a: 1, b: 2})
["a", "b"]
DESTRUCTURING
const {a} = {a: 1}
variable a is set to 1
DESTRUCTURING II
const a = 1
const obj = {a}
variable obj is set to {a: 1}
the DOM
For every HTML tag there is a JavaScript DOM node
CREATE ELEMENT
document.createElement('div')
SET STYLE
<Node>.style.color = "blue"
ADD CLASS
<Node>.classList.add(".myClass")
INNER HTML
<Node>.innerHTML = "<div>hey</div>"
<Node>.innerText = "hey"
ADD CHILD
<Node1>.appendChild(<Node2>)
QUERY SELECTOR
document
.querySelector("#my-id")
QUERY SELECTOR ALL
document
.querySelectorAll(".my-class")
ADD EVENT LISTENER
<Node>.addEventListener("click",
function() {...}
)
Async Programming
Usually network requests, these functions happen outside of the normal "flow" of code
FETCH
fetch('https://google.com')
PROMISE.THEN
.then(result => console.log(result))
THEN CHAINING
.then(...).then(...)
PROMISE.CATCH
.catch(err => console.error(err))
PROMISE.ALL
Promise.all([fetch(...),fetch(...)])
.then(allResults => ...)
ASYNC/AWAIT
const res = await fetch(URL)
ASYNC/AWAIT II
const getURL = async (URL) => {
await fetch(URL)
}
Common Mistakes
Easy mistakes to avoid when writing JavaScript
EQUALS SIGNS
// = is for setting values
let x = 5
// == checks if values are equal
"5" == 5 // true
// === checks if values AND types are equal
"5" === 5 // false
VAR VS LET
if (true) {
var x = "I exist everywhere"
let y = "I only exist in here"
}
console.log(x) // works
console.log(y) // error!
ARRAY CLEARING
const arr = [1, 2, 3]
arr.length = 0 // Oops! Empties the array
console.log(arr) // []
DECIMAL MATH
0.1 + 0.2 === 0.3 // false!
0.1 + 0.2 // gives 0.30000000000000004
TRY/CATCH
try {
riskyOperation()
} catch (error) {
console.error(error)
} finally {
cleanup()
}
Classes & OOP
Object-oriented programming in JavaScript
CLASS BASICS
class Dog {
constructor(name) {
this.name = name
}
bark() {
return `${this.name} says woof!`
}
}
INHERITANCE
class Puppy extends Dog {
constructor(name) {
super(name)
}
playful() {
return `${this.name} wants to play!`
}
}
Modern Features
ES6+ JavaScript features you should know
SPREAD OPERATOR (...)
const arr = [1, 2]
const newArr = [...arr, 3]
const obj = {a: 1}
const newObj = {...obj, b: 2}
NULLISH COALESCING (??)
const value = null ?? 'default'
const zero = 0 ?? 'default'
'default'
0
OPTIONAL CHAINING (?.)
const value = obj?.deep?.property
NPM & Node.js
Managing projects and packages in JavaScript
NPM BASICS
npm init // start a new project
npm install package // add a package
npm start // run your app
npm run dev // start dev mode
PACKAGE.JSON
{
"dependencies": {
"react": "^18.0.0"
},
"scripts": {
"start": "node index.js"
}
}
NODE_MODULES
const express = require('express')
// or
import express from 'express'
Popular Frameworks
Most-used tools for building modern websites
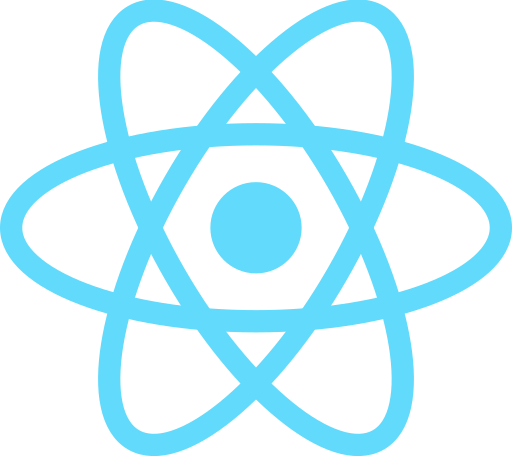
REACT
Made by Facebook
Pros
- •Huge community & job market
- •Flexible and powerful
- •Great for large applications
Cons
- •Steeper learning curve
- •Requires additional libraries
- •Can be overwhelming for beginners
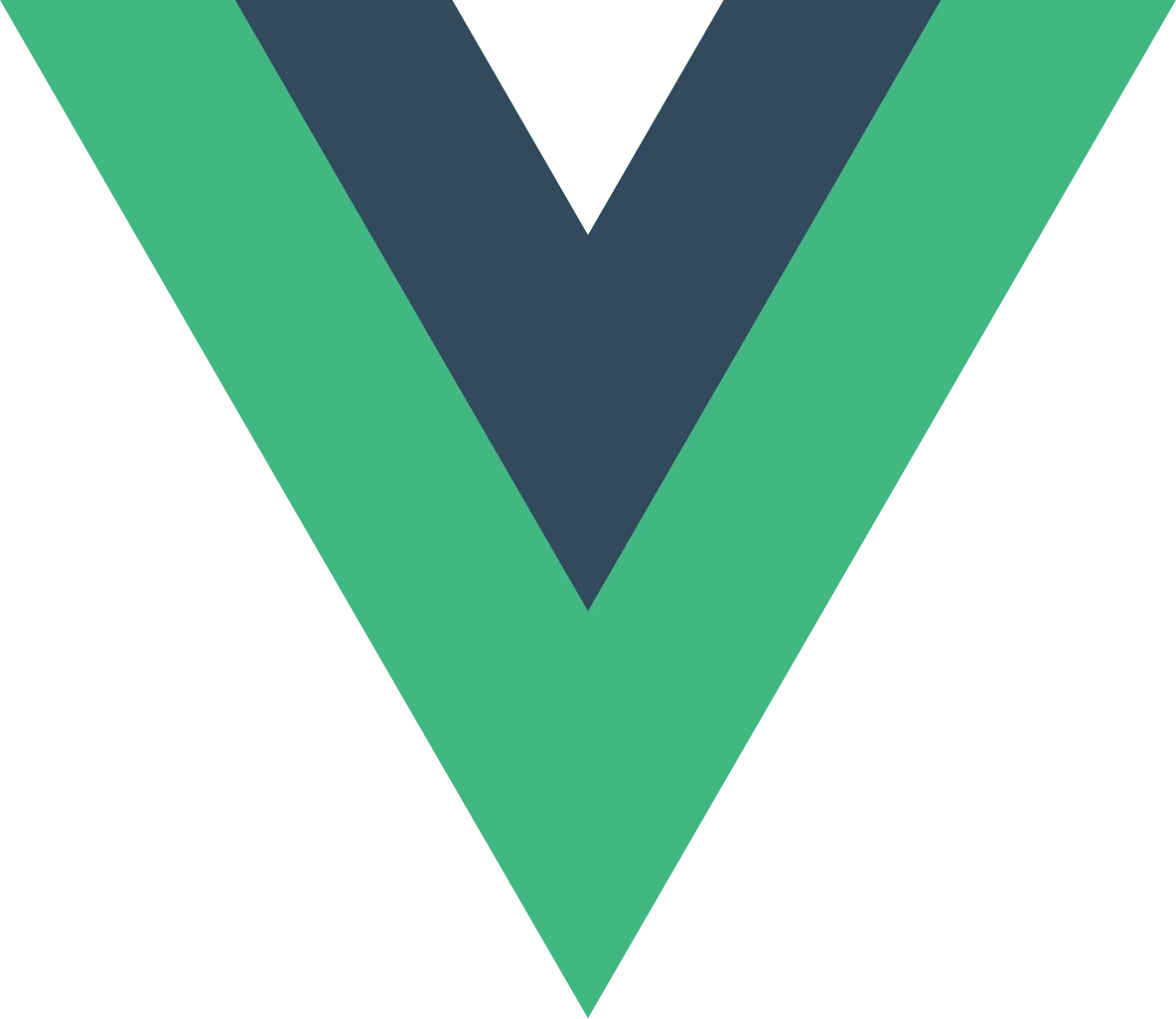
VUE
Community-driven
Pros
- •Easier to learn
- •Great documentation
- •More structured than React
Cons
- •Smaller community than React
- •Fewer job opportunities
- •Less ecosystem support
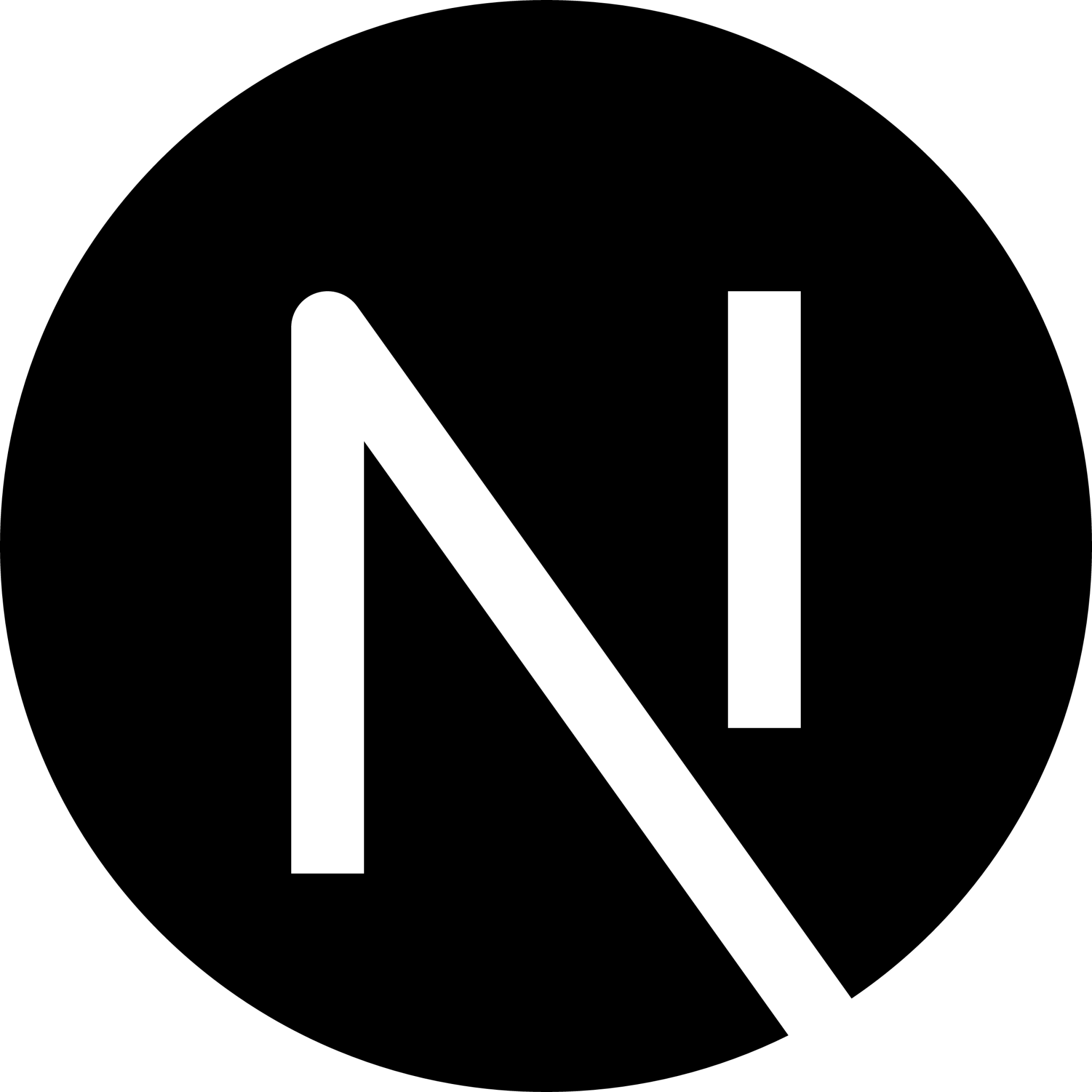
NEXT.JS
Made by Vercel
Pros
- •Built-in routing & optimization
- •Server-side rendering
- •Great developer experience
Cons
- •React knowledge required
- •More complex deployment
- •Overkill for simple sites
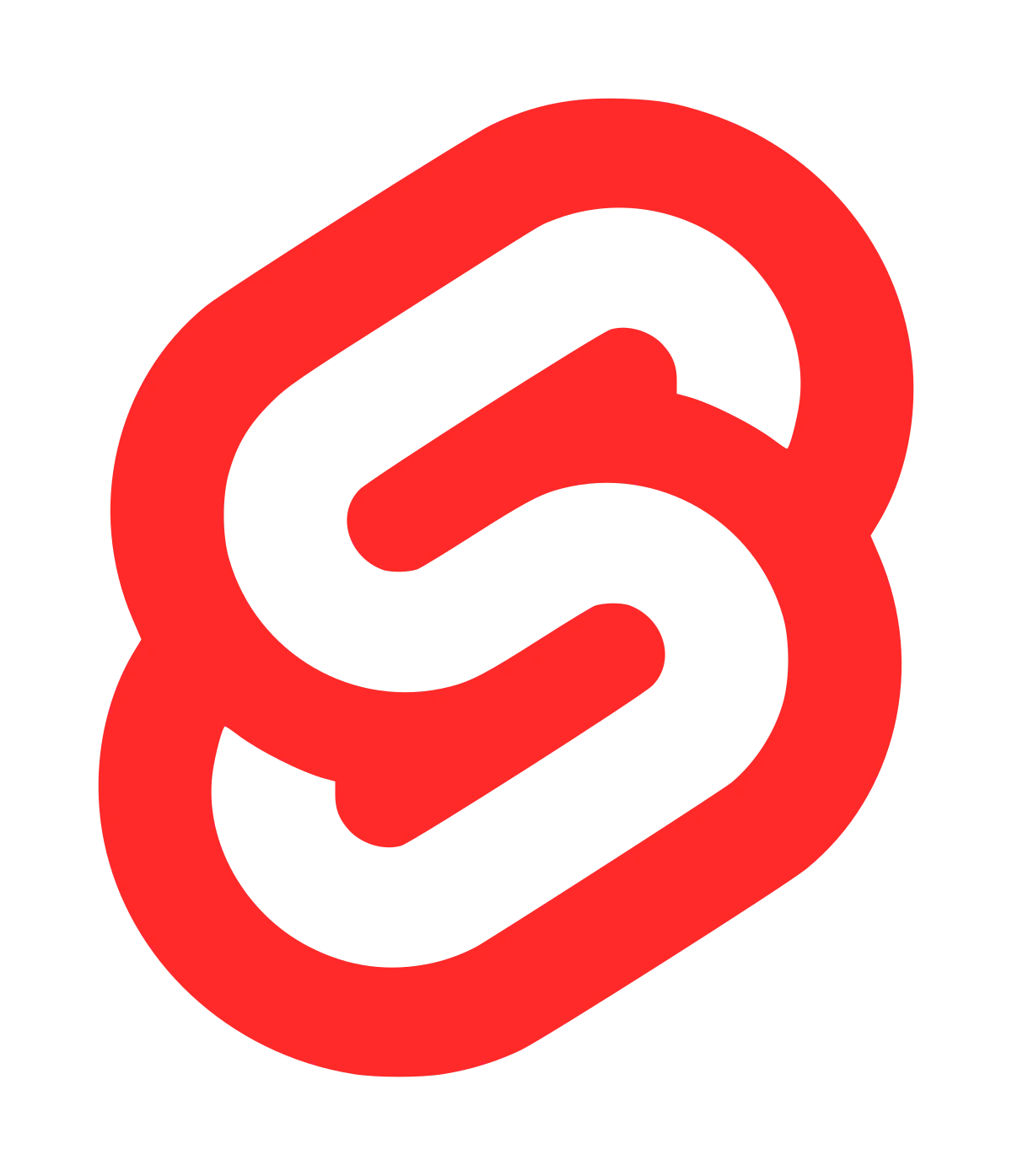
SVELTE
Created by Rich Harris
Pros
- •Very fast performance
- •Simple, clean syntax
- •Small bundle sizes
Cons
- •Smaller ecosystem
- •Fewer learning resources
- •Less mature than others