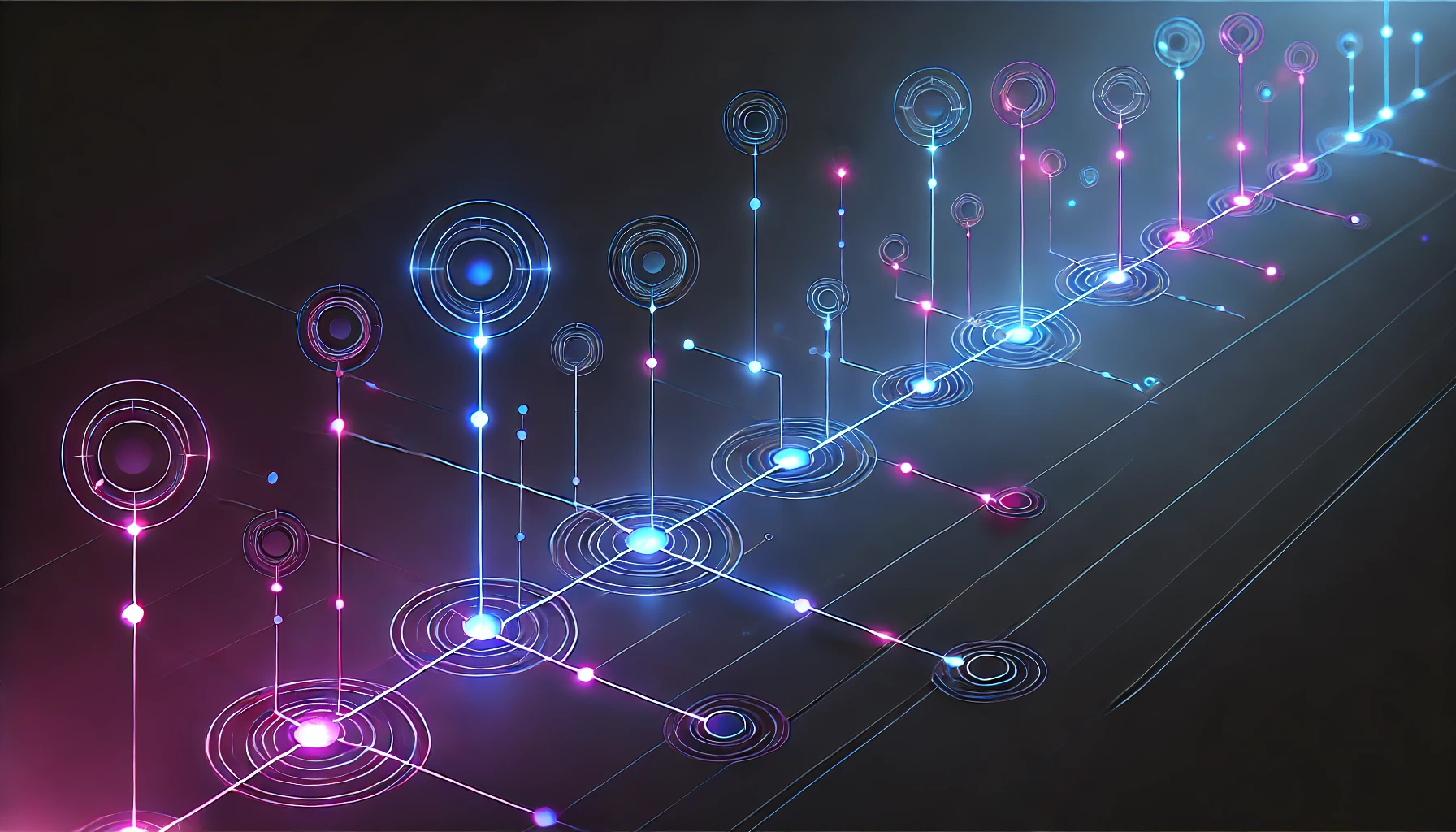
Don't ForgetGit
Cheat Sheet
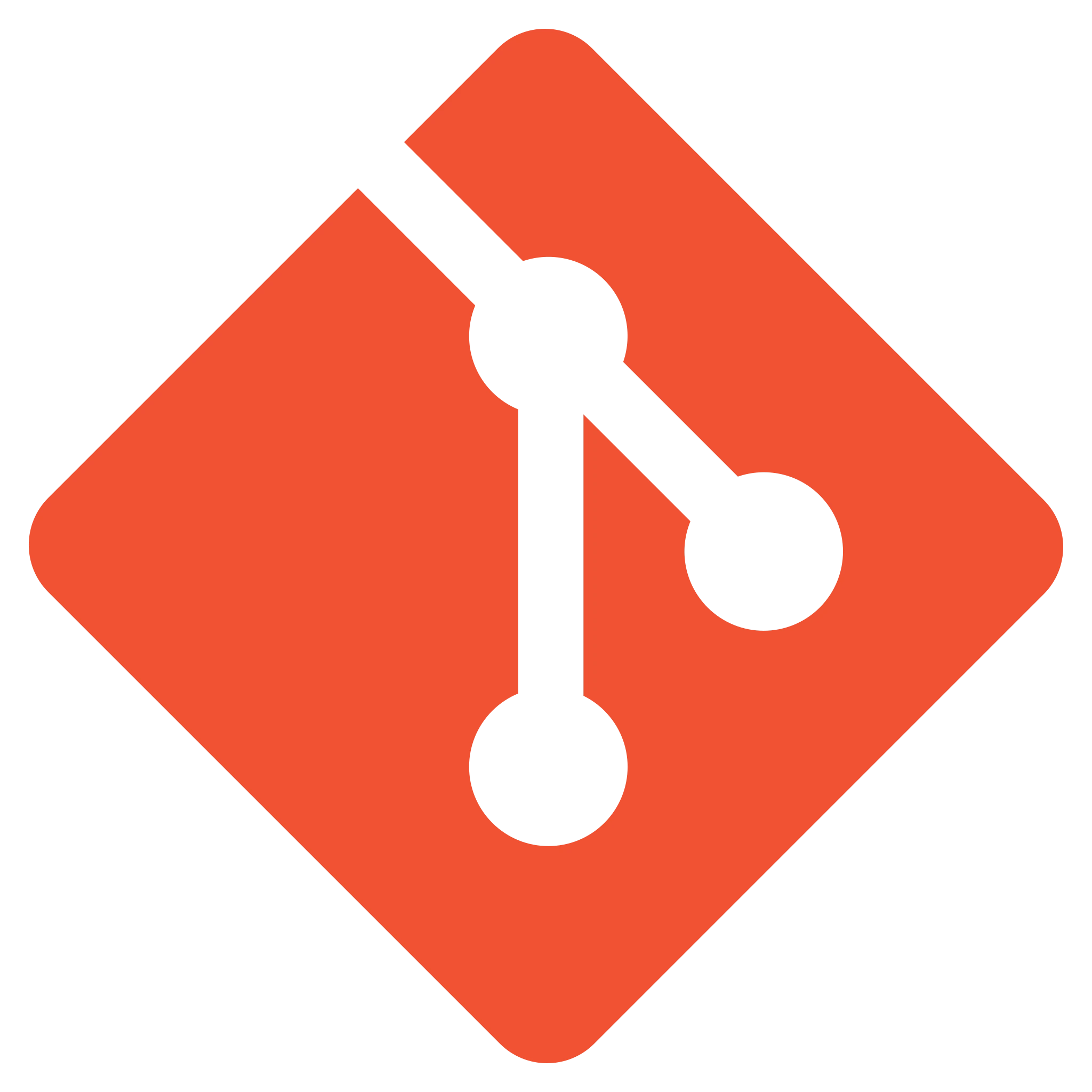
Why Git?
Git Basics
Collaboration & Tools
Best Practices & Troubleshooting
Why Use Git?
Understanding Git's importance
INDUSTRY STANDARD
// Git Market Share 2024
Git: 94.5%
SVN: 4.7%
Others: 0.8%
// GitHub Users: 100M+
// GitLab Users: 30M+
// Bitbucket Users: 10M+
Git is the most widely used version control system, essential for modern software development
KEY BENEFITS
// Version Control
track all changes
revert to any point
// Collaboration
multiple developers
parallel work
// Backup
distributed copies
cloud storage
// Code Review
pull requests
code quality
Git provides robust version control, enables team collaboration, ensures code backup, and facilitates code review
Git vs GitHub
Understanding the difference
GIT
// Git is:
- Version control system
- Runs locally
- Free and open source
- Created by Linus Torvalds
// Core Features:
- Track changes
- Branch and merge
- Local repository
Git is the version control system itself, running on your local machine
GITHUB
// GitHub is:
- Cloud hosting service
- Web-based platform
- Owned by Microsoft
- Built on top of Git
// Added Features:
- Remote storage
- Issue tracking
- Pull requests
- Actions/CI/CD
- Team management
GitHub is a web platform that provides hosting for Git repositories with additional collaboration features
GitHub Alternatives
Other Git hosting platforms
GITLAB
// GitLab Features
- Self-hosted option
- Built-in CI/CD
- Container registry
- Project management
// Best for:
- Enterprise teams
- DevOps pipelines
- Private hosting
GitLab offers robust DevOps features and can be self-hosted
BITBUCKET
// Bitbucket Features
- Atlassian integration
- Jira connection
- Free private repos
- Built-in CI/CD
// Best for:
- Atlassian users
- Small teams
- Private projects
Bitbucket integrates well with other Atlassian products
Setup & Configuration
Get started with Git
CONFIGURATION
# Set your identity
git config --global user.name "John Doe"
git config --global user.email "[email protected]"
# Set default editor
git config --global core.editor "code --wait"
# View settings
git config --list
# Set default branch name
git config --global init.defaultBranch main
# Set line ending preferences
git config --global core.autocrlf true # Windows
git config --global core.autocrlf input # Mac/Linux
Configure Git with your identity and preferences
INITIALIZE REPOSITORY
# Create new repository
git init
# Clone existing repository
git clone https://github.com/user/repo.git
# Clone specific branch
git clone -b branch-name https://github.com/user/repo.git
# Clone with submodules
git clone --recurse-submodules https://github.com/user/repo.git
Start a new repository or clone an existing one
Basic Commands
Essential Git commands
STATUS & STAGING
# Check status
git status
# Add files to staging
git add filename.txt # Single file
git add . # All files
git add *.js # All JS files
git add -p # Interactive staging
# Remove files
git rm filename.txt # Remove from Git and disk
git rm --cached file.txt # Remove from Git only
# Move/rename files
git mv old.txt new.txt
Check repository status and stage changes
COMMITTING
# Commit changes
git commit -m "Commit message"
# Add and commit in one step
git commit -am "Add and commit"
# Amend last commit
git commit --amend
# Empty commit
git commit --allow-empty -m "Empty commit"
# Sign commit
git commit -S -m "Signed commit"
Record changes to the repository
Branch Management
Work with Git branches
BRANCH OPERATIONS
# List branches
git branch # Local branches
git branch -r # Remote branches
git branch -a # All branches
# Create branch
git branch branch-name
git checkout -b branch-name # Create and checkout
# Switch branches
git checkout branch-name
git switch branch-name # New syntax
# Delete branch
git branch -d branch-name # Safe delete
git branch -D branch-name # Force delete
Create and manage branches
ADVANCED BRANCHING
# Create branch from commit
git branch branch-name commit-hash
# Track remote branch
git checkout --track origin/branch-name
# Set upstream branch
git branch -u origin/branch-name
# Rename branch
git branch -m old-name new-name
# List merged/unmerged branches
git branch --merged
git branch --no-merged
Advanced branch operations
Merging & Rebasing
Combine branch changes
MERGING
# Merge branch
git merge branch-name
# Merge with commit
git merge --no-ff branch-name
# Abort merge
git merge --abort
# Continue merge after conflicts
git merge --continue
# Squash merge
git merge --squash branch-name
Merge branches together
REBASING
# Basic rebase
git rebase main
# Interactive rebase
git rebase -i HEAD~3
# Abort rebase
git rebase --abort
# Continue rebase
git rebase --continue
# Skip commit during rebase
git rebase --skip
# Common rebase commands:
# p, pick = use commit
# r, reword = use commit, edit message
# e, edit = use commit, stop for amending
# s, squash = use commit, meld into previous
# f, fixup = like squash, discard message
# d, drop = remove commit
Reapply commits on top of another base
Cherry Picking
Copy specific commits
CHERRY PICK
# Copy a commit to current branch
git cherry-pick commit-hash
# Copy multiple commits
git cherry-pick hash1 hash2
# Copy without committing
git cherry-pick -n commit-hash
Cherry-pick copies specific commits to your current branch
Advanced Rebasing
Clean up history
INTERACTIVE REBASE
# Start interactive rebase
git rebase -i HEAD~3
# Common Commands
pick → keep commit
reword → change message
squash → combine with previous
fixup → combine (discard message)
drop → remove commit
Interactive rebase lets you modify your commit history
Remote Repositories
Work with remote repos
REMOTE MANAGEMENT
# List remotes
git remote -v
# Add remote
git remote add origin https://github.com/user/repo.git
# Remove remote
git remote remove origin
# Change remote URL
git remote set-url origin new-url
# Rename remote
git remote rename old-name new-name
# Show remote info
git remote show origin
Manage remote repositories
SYNCING
# Fetch updates
git fetch origin
git fetch --all
# Pull changes
git pull origin main
git pull --rebase origin main
# Push changes
git push origin main
git push -u origin main # Set upstream
git push --force # Force push (careful!)
git push --force-with-lease # Safer force push
Sync with remote repositories
Collaboration
Work with others
PULL REQUESTS
# Create PR branch
git checkout -b feature-branch
# Push to remote
git push -u origin feature-branch
# Update PR after review
git add .
git commit -m "Address review comments"
git push
# Update PR branch with main
git fetch origin
git rebase origin/main
git push --force-with-lease
Work with pull requests
Git GUIs
Visual Git clients
POPULAR CLIENTS
// GitKraken
- Beautiful interface
- Cross-platform
- Visual commit graph
// Sourcetree
- Free for Atlassian
- Windows/Mac only
- Built-in Git-flow
// GitHub Desktop
- Simple interface
- GitHub integration
- Perfect for beginners
GUI clients make Git more visual and easier to use
Git Aliases
Create custom shortcuts
CREATING ALIASES
# Add aliases to .gitconfig
git config --global alias.co checkout
git config --global alias.br branch
git config --global alias.ci commit
git config --global alias.st status
# Create complex alias
git config --global alias.lg "log --graph --oneline"
Aliases create shortcuts for frequently used commands
Writing Commit Messages
Best practices for clear commits
MESSAGE STRUCTURE
// Format:
<type>(<scope>): <subject>
<body>
<footer>
// Examples:
feat(auth): add login functionality
fix(api): handle timeout errors
docs(readme): update installation steps
Follow conventional commits format for clear, consistent messages
BEST PRACTICES
// DO:
- Use imperative mood
- Keep subject under 50 chars
- Capitalize first letter
- No period at end
// DON'T:
- Don't use past tense
- No 'fixed', use 'fix'
- No vague messages
- No long subjects
Write clear, concise commit messages that explain what and why (not how)
Why Branches?
Understanding branching strategies
WHY BRANCH?
// Benefits of Branching
- Isolate new features
- Work in parallel
- Stable main branch
- Easy rollback
- Clean testing
// Common Branches
main → production code
develop → next release
feature/ → new features
hotfix/ → urgent fixes
Branches allow parallel development and protect stable code
GIT FLOW
// Git Flow Strategy
main → production
develop → development
feature/ → new features
release/ → release prep
hotfix/ → urgent fixes
// Feature Branch Flow
1. Branch from develop
2. Work on feature
3. Create pull request
4. Review and merge
Git Flow is a popular branching strategy for managing releases
Common Git Issues
Solutions to frequent problems
MERGE CONFLICTS
# Check conflict status
git status
# Abort merge
git merge --abort
# After fixing conflicts
git add .
git commit -m "Resolve conflicts"
# Prevent conflicts
git pull --rebase origin main
Handle and prevent common merge conflicts
FIXING MISTAKES
# Undo last commit
git reset --soft HEAD~1
# Undo staged changes
git reset HEAD file.txt
# Discard local changes
git checkout -- file.txt
# Reset to remote
git fetch origin
git reset --hard origin/main
Common commands to fix Git mistakes